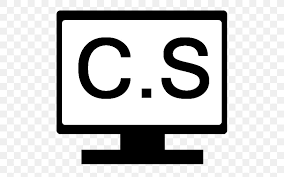
OVERVIEW
The objective is to write a function which can identify the longest substring in a string which does not have repeating characters. Examples given below:
String “abcabcbb” . The answer would be 3 because the substring is abc
String “bbbbb”. The answer would be 1 because the substring is b
String “pwwkew”. The answer would be 3 because the substring is wke
SAMPLE CODE
class Solution {
public int lengthOfLongestSubstring(String s) {
int longestStart = -1;
int longestLength = -1;
int max = s.length();
if (max < 1)
return 0;
if (max < 2)
return 1;
StringBuilder sb = new StringBuilder("");
int length = 0;
int counter = 0;
int startedAt = counter;
while (counter < max) {
String check = s.substring(counter, counter+1);
if (sb.toString().indexOf(check) == -1) {
sb.append(check);
length++;
if (length > longestLength) {
longestLength = length;
longestStart = counter;
}
counter++;
} else {
sb = new StringBuilder("");
length = 0;
startedAt ++;
counter = startedAt;
}
}
return longestLength;
}
public static void main(String[] args) {
System.out.println(lengthOfLongestSubstring("pwwkew"));
}
}
The output is given below:
3
Leave a Reply