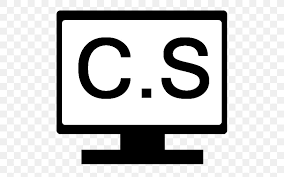
OVERVIEW
We see how to rotate elements in an array both right and left a certain number of times. We use the mod operator to cut down the number of iterations specified. For eg.if there are 5 elements in an array and we are required to rotate it 5 times then it is the same as not rotating it at all. Likewise if we need to rotate it 6 times then it is the same as rotating it just once.
SAMPLE CODE TO ROTATE RIGHT
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Algorithms2
{
internal class Program
{
static void Main(string[] args)
{
int[] nums = { 5, 6, 7, 8, 9, 10, 11, 12, 13 };
int rotate = 2;
Console.WriteLine("");
foreach(int num in nums)
{
Console.Write(num + ",");
}
Console.WriteLine(" rotate " + rotate + " times.");
int[] nums2 = rotateRight(nums, rotate);
Console.WriteLine("");
foreach (int num in nums2)
{
Console.Write(num + ",");
}
Console.WriteLine("");
}
static int[] rotateRight(int[] nums, int k)
{
int maxElems = nums.Length;
if (maxElems < 2)
return nums;
k = k % maxElems;
for (int steps = 0; steps < k; steps++)
{
int temp = nums[maxElems - 1];
for (int i = maxElems - 2; i >= 0; i--)
{
nums[i + 1] = nums[i];
}
nums[0] = temp;
}
return nums;
}
}
}
The output is shown below:
5,6,7,8,9,10,11,12,13, rotate 2 times. 12,13,5,6,7,8,9,10,11, Press any key to continue . . .
SAMPLE CODE TO ROTATE LEFT
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Algorithms2
{
internal class Program
{
static void Main(string[] args)
{
int[] nums = { 5, 6, 7, 8, 9, 10, 11, 12, 13 };
int rotate = 2;
Console.WriteLine("");
foreach(int num in nums)
{
Console.Write(num + ",");
}
Console.WriteLine(" rotate " + rotate + " times.");
int[] nums2 = rotateLeft(nums, rotate);
Console.WriteLine("");
foreach (int num in nums2)
{
Console.Write(num + ",");
}
Console.WriteLine("");
}
static int[] rotateLeft(int[] nums, int k)
{
int maxElems = nums.Length;
if (maxElems < 2)
return nums;
k = k % maxElems;
for (int steps = 0; steps < k; steps++)
{
int temp = nums[0];
for (int i = 1; i <= maxElems-1; i++)
{
nums[i-1] = nums[i];
}
nums[maxElems-1] = temp;
}
return nums;
}
}
}
The output is shown below:
5,6,7,8,9,10,11,12,13, rotate 2 times. 7,8,9,10,11,12,13,5,6, Press any key to continue . . .
Leave a Reply