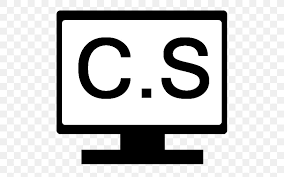
OVERVIEW
The code given below prints all the possible permutations of a string. It does not check for duplicate occurrences of a character.
SAMPLE CODE
public class StringPermutation {
public void permute(String str, int start, int stop) {
if (stop == start) {
System.out.println(str);
return;
}
for(int i=start;i <= stop; i++) {
str = swap(str, start, i);
permute(str, start+1, stop);
}
}
public String swap(String str, int a, int b) {
char[] chars = str.toCharArray();
char temp = chars[a];
chars[a] = chars[b];
chars[b] = temp;
return String.valueOf(chars);
}
public static void main(String args[]) {
StringPermutation perm = new StringPermutation();
if (args.length < 1) {
System.out.println("Needs string argument");
return;
}
String str = args[0];
System.out.println("Processing " + str + " of length " + str.length());
perm.permute(str, 0, str.length()-1);
}
}
The output is given below:
amit@amit-viao:/var/projects/java$ java StringPermutation abc Processing abc of length 3 abc acb bac bca cba cab
Leave a Reply