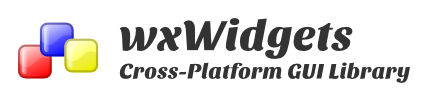
OVERVIEW
wxGridBagSizer adds more complexity to the wxFlexGridSizer by allowing controls to span multiple rows or columns. We can even specify which row or column to place a control in. This manipulation of placements can be done at runtime as well.
The constructor of wxGridBagSizer does not specify the number of rows or columns. It only specifies the horizontal and vertical gap between the cells.
The sample code below replicates the same window as in wxFlexGridSizer but using wxGridBagSizer
SAMPLE CODE
gridbagsizer.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
#include <wx/gbsizer.h>
class GridBagSizer: public wxFrame {
public:
GridBagSizer(const wxString &title);
};
gridbagsizer.cpp
#include "gridbagsizer.h"
GridBagSizer::GridBagSizer(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,250)) {
wxPanel *basePanel = new wxPanel(this,-1);
wxGridBagSizer *gbs = new wxGridBagSizer(3,4);
gbs->SetEmptyCellSize(wxSize(20,10));
basePanel->SetSizer(gbs);
wxStaticText *lblA1= new wxStaticText(basePanel, -1, wxT("Answer 1"));
wxStaticText *lblA2= new wxStaticText(basePanel, -1, wxT("Answer 2"));
wxStaticText *lblA3= new wxStaticText(basePanel, -1, wxT("Answer 3"));
wxStaticText *lblA4= new wxStaticText(basePanel, -1, wxT("Answer 4"));
wxTextCtrl *txtA1= new wxTextCtrl(basePanel, -1, wxT(""));
wxTextCtrl *txtA2= new wxTextCtrl(basePanel, -1, wxT(""));
wxTextCtrl *txtA3= new wxTextCtrl(basePanel, -1, wxT(""));
wxTextCtrl *txtA4= new wxTextCtrl(basePanel, -1, wxT(""), wxPoint(-1,-1), wxSize(-1,-1), wxTE_MULTILINE);
gbs->Add(lblA1, wxGBPosition(0,0), wxGBSpan(1,1), wxEXPAND);
gbs->Add(lblA2, wxGBPosition(1,0), wxGBSpan(1,1), wxEXPAND);
gbs->Add(lblA3, wxGBPosition(2,0), wxGBSpan(1,1), wxEXPAND);
gbs->Add(lblA4, wxGBPosition(3,0), wxGBSpan(1,1), wxEXPAND);
gbs->Add(txtA1, wxGBPosition(0,1), wxGBSpan(1,4), wxEXPAND);
gbs->Add(txtA2, wxGBPosition(1,1), wxGBSpan(1,4), wxEXPAND);
gbs->Add(txtA3, wxGBPosition(2,1), wxGBSpan(1,4), wxEXPAND);
gbs->Add(txtA4, wxGBPosition(3,1), wxGBSpan(4,4), wxEXPAND);
gbs->AddGrowableRow(3);
gbs->AddGrowableCol(1);
Center();
}
The output is shown below
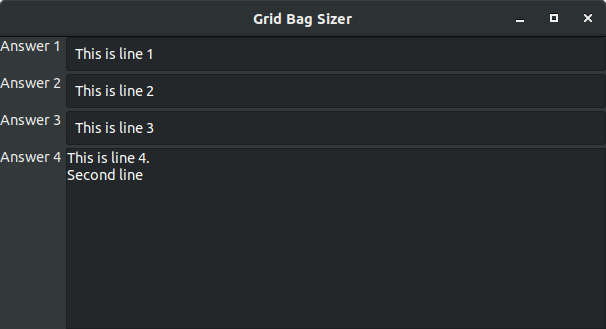
Leave a Reply