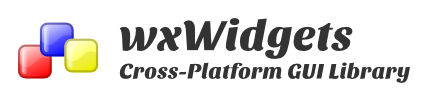
OVERVIEW
Now we can actually start using wxWidgets for building GUI screens. The example code in this section will show how to create windows and panels and use of common controls like labels and buttons. We are not going to look at layout management as that is dealt with in later sections. The objective here is to get familiar with creating basic GUI applications.
If you have followed the posts in the Introduction page on how to setup wxWidgets and run a sample program, then you now know how to build an application which has a single cpp source file.
In later examples in this series, you will have to work with multiple cpp files. We first look at how to build an executable with multiple source files before we go into the windowing examples.
We have two files simple.cpp which is the wxWidget GUI window and main.cpp which is the main wxApp that calls the Window object in simple.cpp. As per standard C++ practice , we define header files for each class.
SAMPLE CODE
simple.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Simple: public wxFrame {
public:
Simple(const wxString& title);
};
simple.cpp
#include "simple.h"
Simple::Simple(const wxString& title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(700,300)) {
Centre();
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class MyApp: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "simple.h"
IMPLEMENT_APP(MyApp)
bool MyApp::OnInit() {
Simple *simple = new Simple(wxT("Simple Window test"));
simple->Show(true);
return true;
}
To build the executable under Linux use the command
g++ main.cpp simple.cpp ``wx-config --cxxflags --libs
`` -o simple
Use only single surrounding backticks in the command above and not the double backticks as shown .This will create an executable called simple which you can run via ./simple
To build the executable under Windows , make the following changes to the sample makefile from the earlier post:
# =========================================================================
# This makefile was generated by
# Bakefile 0.2.12 (http://www.bakefile.org)
# Do not modify, all changes will be overwritten!
# =========================================================================
prefix = /mingw64
exec_prefix = ${prefix}
datarootdir = ${prefix}/share
INSTALL = /usr/bin/install -c
EXEEXT = .exe
WINDRES = windres
NM = nm
BK_DEPS = /d/wxWidgets/build-debug/bk-deps
srcdir = .
top_srcdir = ..
LIBS = -lz -lrpcrt4 -loleaut32 -lole32 -luuid -llzma -luxtheme -lwinspool -lwinmm -lshell32 -lshlwapi -lcomctl32 -lcomdlg32 -ladvapi32 -lversion -lwsock32 -lgdi32 -loleacc -lwinhttp
LDFLAGS_GUI = -mwindows
CXX = g++
CXXFLAGS =
CPPFLAGS =
LDFLAGS =
USE_DPI_AWARE_MANIFEST = 2
WX_LIB_FLAVOUR =
TOOLKIT = MSW
TOOLKIT_LOWERCASE = msw
TOOLKIT_VERSION =
TOOLCHAIN_FULLNAME = msw-unicode-static-3.1
EXTRALIBS = -lz -lrpcrt4 -loleaut32 -lole32 -luuid -llzma -luxtheme -lwinspool -lwinmm -lshell32 -lshlwapi -lcomctl32 -lcomdlg32 -ladvapi32 -lversion -lwsock32 -lgdi32 -loleacc -lwinhttp
EXTRALIBS_XML = -lexpat
EXTRALIBS_GUI = -llzma
WX_CPPFLAGS = -I${wx_top_builddir}/lib/wx/include/msw-unicode-static-3.1 -I${top_srcdir}/include -D_FILE_OFFSET_BITS=64
WX_CXXFLAGS = -Wall -Wundef -Wunused-parameter -Wno-ctor-dtor-privacy -Woverloaded-virtual -g -O0
WX_LDFLAGS =
HOST_SUFFIX =
SAMPLES_RPATH_FLAG =
SAMPLES_CXXFLAGS =
wx_top_builddir = D:/wxWidgets/build-debug
### Variables: ###
DESTDIR =
WX_RELEASE = 3.1
WX_VERSION = $(WX_RELEASE).5
LIBDIRNAME = $(wx_top_builddir)/lib
MAINAPP_CXXFLAGS = $(WX_CPPFLAGS) -D__WX$(TOOLKIT)__ $(__WXUNIV_DEFINE_p) \
$(__DEBUG_DEFINE_p) $(__EXCEPTIONS_DEFINE_p) $(__RTTI_DEFINE_p) \
$(__THREAD_DEFINE_p) -I$(srcdir) $(__DLLFLAG_p) -I$(srcdir). \
$(WX_CXXFLAGS) $(CPPFLAGS) $(CXXFLAGS)
MAINAPP_OBJECTS = \
$(__MAINAPP___win32rc) \
MAINAPP_main.o \
MAINAPP_simple.o
### Conditionally set variables: ###
#CXXC = $(CXX)
CXXC = $(BK_DEPS) $(CXX)
#PORTNAME = base
PORTNAME = $(TOOLKIT_LOWERCASE)$(TOOLKIT_VERSION)
#WXBASEPORT = _carbon
#WXDEBUGFLAG = d
WXUNICODEFLAG = u
#WXUNIVNAME = univ
EXTRALIBS_FOR_BASE = $(EXTRALIBS)
#EXTRALIBS_FOR_BASE = $(EXTRALIBS) \
# $(EXTRALIBS_XML) $(EXTRALIBS_GUI)
EXTRALIBS_FOR_GUI = $(EXTRALIBS_GUI)
#EXTRALIBS_FOR_GUI =
#__WXUNIV_DEFINE_p = -D__WXUNIVERSAL__
#__WXUNIV_DEFINE_p_1 = --define __WXUNIVERSAL__
#__DEBUG_DEFINE_p = -DwxDEBUG_LEVEL=0
#__DEBUG_DEFINE_p_1 = --define wxDEBUG_LEVEL=0
#__EXCEPTIONS_DEFINE_p = -DwxNO_EXCEPTIONS
#__EXCEPTIONS_DEFINE_p_1 = --define wxNO_EXCEPTIONS
#__RTTI_DEFINE_p = -DwxNO_RTTI
#__RTTI_DEFINE_p_1 = --define wxNO_RTTI
#__THREAD_DEFINE_p = -DwxNO_THREADS
#__THREAD_DEFINE_p_1 = --define wxNO_THREADS
#__DLLFLAG_p = -DWXUSINGDLL
#__DLLFLAG_p_1 = --define WXUSINGDLL
__WIN32_DPI_MANIFEST_p = \
--define \
wxUSE_DPI_AWARE_MANIFEST=$(USE_DPI_AWARE_MANIFEST)
COND_PLATFORM_OS2_1___MAINAPP___os2_emxbindcmd = $(NM) simplewindow$(EXEEXT) \
| if grep -q pmwin.763 ; then emxbind -ep simplewindow$(EXEEXT) ; fi
#__MAINAPP___os2_emxbindcmd = $(COND_PLATFORM_OS2_1___MAINAPP___os2_emxbindcmd)
__RCDEFDIR_p = --include-dir \
$(LIBDIRNAME)/wx/include/$(TOOLCHAIN_FULLNAME)
#__MAINAPP___win32rc = MAINAPP_MAINAPP_rc.o
#__MAINAPP_app_Contents_PkgInfo___depname \
# = simplewindow.app/Contents/PkgInfo
#__MAINAPP_bundle___depname = MAINAPP_bundle
#____MAINAPP_BUNDLE_TGT_REF_DEP = \
# $(__MAINAPP_app_Contents_PkgInfo___depname)
#____MAINAPP_BUNDLE_TGT_REF_DEP \
# = $(__MAINAPP_app_Contents_PkgInfo___depname)
#____MAINAPP_BUNDLE_TGT_REF_DEP \
# = $(__MAINAPP_app_Contents_PkgInfo___depname)
#____MAINAPP_BUNDLE_TGT_REF_DEP \
# = $(__MAINAPP_app_Contents_PkgInfo___depname)
#____MAINAPP_BUNDLE_TGT_REF_DEP = \
# $(__MAINAPP_app_Contents_PkgInfo___depname)
COND_MONOLITHIC_0___WXLIB_CORE_p = \
-lwx_$(PORTNAME)$(WXUNIVNAME)$(WXUNICODEFLAG)$(WXDEBUGFLAG)$(WX_LIB_FLAVOUR)_core-$(WX_RELEASE)$(HOST_SUFFIX)
__WXLIB_CORE_p = $(COND_MONOLITHIC_0___WXLIB_CORE_p)
COND_MONOLITHIC_0___WXLIB_BASE_p = \
-lwx_base$(WXBASEPORT)$(WXUNICODEFLAG)$(WXDEBUGFLAG)$(WX_LIB_FLAVOUR)-$(WX_RELEASE)$(HOST_SUFFIX)
__WXLIB_BASE_p = $(COND_MONOLITHIC_0___WXLIB_BASE_p)
COND_MONOLITHIC_1___WXLIB_MONO_p = \
-lwx_$(PORTNAME)$(WXUNIVNAME)$(WXUNICODEFLAG)$(WXDEBUGFLAG)$(WX_LIB_FLAVOUR)-$(WX_RELEASE)$(HOST_SUFFIX)
#__WXLIB_MONO_p = $(COND_MONOLITHIC_1___WXLIB_MONO_p)
#__LIB_SCINTILLA_IF_MONO_p \
# = \
# -lwxscintilla$(WXDEBUGFLAG)$(WX_LIB_FLAVOUR)-$(WX_RELEASE)$(HOST_SUFFIX)
__LIB_TIFF_p \
= \
-lwxtiff$(WXDEBUGFLAG)$(WX_LIB_FLAVOUR)-$(WX_RELEASE)$(HOST_SUFFIX)
__LIB_JPEG_p \
= \
-lwxjpeg$(WXDEBUGFLAG)$(WX_LIB_FLAVOUR)-$(WX_RELEASE)$(HOST_SUFFIX)
__LIB_PNG_p \
= \
-lwxpng$(WXDEBUGFLAG)$(WX_LIB_FLAVOUR)-$(WX_RELEASE)$(HOST_SUFFIX)
#__LIB_ZLIB_p = \
# -lwxzlib$(WXDEBUGFLAG)$(WX_LIB_FLAVOUR)-$(WX_RELEASE)$(HOST_SUFFIX)
COND_wxUSE_REGEX_builtin___LIB_REGEX_p = \
-lwxregex$(WXUNICODEFLAG)$(WXDEBUGFLAG)$(WX_LIB_FLAVOUR)-$(WX_RELEASE)$(HOST_SUFFIX)
__LIB_REGEX_p = $(COND_wxUSE_REGEX_builtin___LIB_REGEX_p)
#__LIB_EXPAT_p = \
# -lwxexpat$(WXDEBUGFLAG)$(WX_LIB_FLAVOUR)-$(WX_RELEASE)$(HOST_SUFFIX)
### Targets: ###
all: mainapp$(EXEEXT) $(__MAINAPP_bundle___depname)
install:
uninstall:
install-strip: install
clean:
rm -rf ./.deps ./.pch
rm -f ./*.o
rm -f mainapp$(EXEEXT)
rm -rf mainapp.app
distclean: clean
rm -f config.cache config.log config.status bk-deps bk-make-pch shared-ld-sh Makefile
mainapp$(EXEEXT): $(MAINAPP_OBJECTS)
$(CXX) -o $@ $(MAINAPP_OBJECTS) -L$(LIBDIRNAME) $(LDFLAGS_GUI) $(LDFLAGS) $(WX_LDFLAGS) $(__WXLIB_CORE_p) $(__WXLIB_BASE_p) $(__WXLIB_MONO_p) $(__LIB_SCINTILLA_IF_MONO_p) $(__LIB_TIFF_p) $(__LIB_JPEG_p) $(__LIB_PNG_p) $(EXTRALIBS_FOR_GUI) $(__LIB_REGEX_p) $(__LIB_EXPAT_p) $(EXTRALIBS_FOR_BASE) $(LIBS)
$(__MAINAPP___os2_emxbindcmd)
#simplewindow.app/Contents/PkgInfo: simplewindow$(EXEEXT) $(top_srcdir)/src/osx/carbon/Info.plist.in $(top_srcdir)/src/osx/carbon/wxmac.icns
# mkdir -p simplewindow.app/Contents
# mkdir -p simplewindow.app/Contents/MacOS
# mkdir -p simplewindow.app/Contents/Resources
#
#
# sed -e "s/IDENTIFIER/`echo $(srcdir) | sed -e 's,\.\./,,g' | sed -e 's,/,.,g'`/" \
# -e "s/EXECUTABLE/simplewindow/" \
# -e "s/VERSION/$(WX_VERSION)/" \
# $(top_srcdir)/src/osx/carbon/Info.plist.in >simplewindow.app/Contents/Info.plist
#
#
# /bin/echo "APPL????" >simplewindow.app/Contents/PkgInfo
#
#
# ln -f simplewindow$(EXEEXT) simplewindow.app/Contents/MacOS/simplewindow
#
#
# cp -f $(top_srcdir)/src/osx/carbon/wxmac.icns simplewindow.app/Contents/Resources/wxmac.icns
#MAINAPP_bundle: $(____MAINAPP_BUNDLE_TGT_REF_DEP)
#
#MAINAPP_MAINAPP_rc.o: $(srcdir)/main.rc
# $(WINDRES) -i$< -o$@ --define __WX$(TOOLKIT)__ $(__WXUNIV_DEFINE_p_1) $(__DEBUG_DEFINE_p_1) $(__EXCEPTIONS_DEFINE_p_1) $(__RTTI_DEFINE_p_1) $(__THREAD_DEFINE_p_1) --include-dir $(srcdir) $(__DLLFLAG_p_1) $(__WIN32_DPI_MANIFEST_p) --include-dir $(srcdir). $(__RCDEFDIR_p) --include-dir $(top_srcdir)/include
MAINAPP_simple.o: $(srcdir)/simple.cpp
$(CXXC) -c -o $@ $(MAINAPP_CXXFLAGS) $(srcdir)/simple.cpp
MAINAPP_main.o: $(srcdir)/main.cpp
$(CXXC) -c -o $@ $(MAINAPP_CXXFLAGS) $(srcdir)/main.cpp
# Include dependency info, if present:
-include ./.deps/*.d
.PHONY: all install uninstall clean distclean MAINAPP_bundle
Run the makefile within MSYS2 MingW64-bit console by typing
make
On successful completion run the executable from within MSYS2 console with ./simple
If you want to run it from outside the MSYS console, make sure you have the supporting dlls in place and double click simple.exe
This is how the app will look under Ubuntu with a Dark theme:
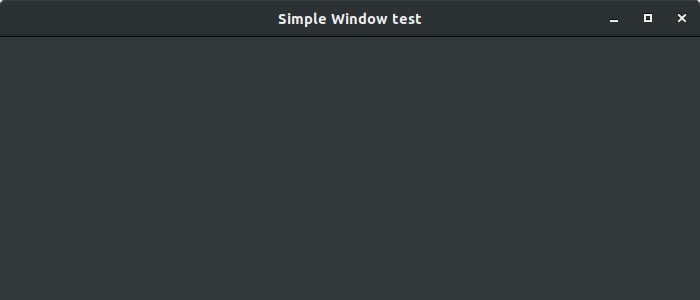
The following topics are covered further in this section:
- 3a. Simple Window
- 3b. Application Icon
- 3c. Buttons & Labels
- 3d. Panels
- 3e Preview to Event Handling
Leave a Reply