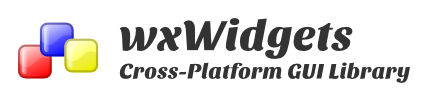
All standard file and directory operations are supported in wxWidgets. Below are two sample programs – the first writes and reads a text file while the second does directory functions.
SAMPLE CODE
filereadwrite.cpp
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
#include <wx/string.h>
#include <wx/file.h>
#include <wx/textfile.h>
int main(int argc, char **argv) {
wxInitialize();
wxString line = wxT("This is a line of text\nThis is second line.\nThis is last line.\n");
wxFile file;
file.Create(wxT("file.dat"), true);
if (file.IsOpened()) {
file.Write(line);
file.Close();
} else {
wxPuts(wxT("Could not open file"));
return -1;
}
wxTextFile tfile;
tfile.Open(wxT("file.dat"));
if (!tfile.IsOpened()) {
wxPuts("File could not be read");
return -1;
}
wxPrintf(wxT("Line count: %ld\n"), tfile.GetLineCount());
wxString data;
data = tfile.GetFirstLine();
while (!tfile.Eof()) {
wxPuts(data);
data = tfile.GetNextLine();
}
tfile.Close();
wxUninitialize();
}
The output is given below:
Line count: 3 This is a line of text This is second line. This is last line.
directory.cpp
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
#include <wx/string.h>
#include <wx/filefn.h>
#include <wx/dir.h>
int main(int argc, char **argv) {
wxInitialize();
wxDir curr(wxGetCwd());
wxString f;
bool flag = curr.GetFirst(&f, wxEmptyString, wxDIR_FILES | wxDIR_DIRS);
while (flag) {
wxPuts(f);
flag = curr.GetNext(&f);
}
wxPuts("========================");
flag = curr.GetFirst(&f, wxT("*.cpp"), wxDIR_FILES | wxDIR_DIRS);
while (flag) {
wxPuts(f);
flag = curr.GetNext(&f);
}
wxPuts("========================");
bool ret = wxMkdir(wxT("tempdir"));
if (!ret) {
wxPuts("Could not create tempdir");
} else {
wxPuts("tempdir created");
ret = wxRmdir(wxT("tempdir"));
if (ret)
wxPuts("tempdir deleted");
}
wxUninitialize();
}
The output is given below:
file.dat platform.cpp platform directory filereadwrite directory.cpp console datetime pcontrol pcontrol.cpp strings datetime.cpp filereadwrite.cpp strings.cpp console.cpp platform.cpp directory.cpp pcontrol.cpp datetime.cpp filereadwrite.cpp strings.cpp console.cpp tempdir created tempdir deleted
Leave a Reply