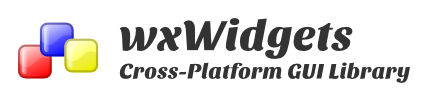
OVERVIEW
wxStaticBoxSizer is the same as wxBoxSizer except it draws a border around the controls contained within it. This is good for grouping controls visually.
SAMPLE CODE
wxstaticboxsizer.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class StaticBoxSizer: public wxFrame {
public:
StaticBoxSizer(const wxString &title);
};
wxstaticboxsizer.cpp
#include "wxstaticboxsizer.h"
StaticBoxSizer::StaticBoxSizer(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,250)) {
wxPanel *basePanel = new wxPanel(this,-1);
wxBoxSizer *vbox = new wxBoxSizer(wxVERTICAL);
basePanel->SetSizer(vbox);
wxPanel *panel1 = new wxPanel(basePanel, -1);
wxButton *btn1 = new wxButton(panel1, -1, wxT("Button 1"));
wxButton *btn2 = new wxButton(panel1, -1, wxT("Button 2"));
wxButton *btn3 = new wxButton(panel1, -1, wxT("Button 3"));
wxStaticBoxSizer *hbox = new wxStaticBoxSizer(wxHORIZONTAL, panel1);
hbox->Add(btn1, 1, wxALL, 10);
hbox->Add(btn2,1, wxALL, 10);
hbox->Add(btn3,1, wxALL, 10);
panel1->SetSizer(hbox);
vbox->Add(panel1, 0, wxALIGN_CENTER);
Center();
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "wxstaticboxsizer.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
StaticBoxSizer *app = new StaticBoxSizer(wxT("Static Box Sizer"));
app->Show(true);
}
The output is shown below
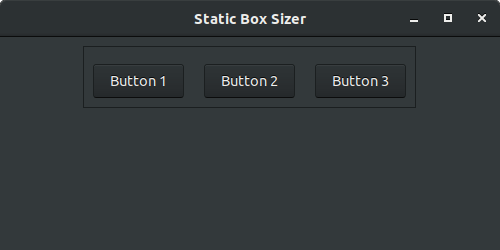
Leave a Reply