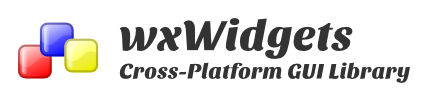
OVERVIEW
wxGridSizer lets you place controls in the form of a grid using rows and columns. All the cells in the grid will have the same size. The constructor method is in the form of wxGridSizer(rows, columns, vertical gap, horizontal gap)
In the sample code below, we will create a mini spreadsheet layout with buttons for the row and column headings and text controls for the actual cells. When you resize the window, the cells will stretch to fill the empty space.
SAMPLE CODE
wxgridsizer.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class GridSizer: public wxFrame {
public:
GridSizer(const wxString &title);
};
wxgridsizer.cpp
#include "wxgridsizer.h"
GridSizer::GridSizer(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,200)) {
wxPanel *basePanel = new wxPanel(this, -1);
wxGridSizer *baseSizer = new wxGridSizer(5,5,0,0);
basePanel->SetSizer(baseSizer);
wxButton *btnHome = new wxButton(basePanel, -1, wxT(""));
baseSizer->Add(btnHome,1, wxEXPAND);
wxButton *btnCol1 = new wxButton(basePanel, -1, wxT("A"));
baseSizer->Add(btnCol1,1, wxEXPAND);
wxButton *btnCol2 = new wxButton(basePanel, -1, wxT("B"));
baseSizer->Add(btnCol2,1, wxEXPAND);
wxButton *btnCol3 = new wxButton(basePanel, -1, wxT("C"));
baseSizer->Add(btnCol3,1, wxEXPAND);
wxButton *btnCol4 = new wxButton(basePanel, -1, wxT("D"));
baseSizer->Add(btnCol4,1, wxEXPAND);
for (int row = 1; row <=4; row++) {
for(int col = 1; col <=5; col++) {
if (col == 1) {
wxButton *col = new wxButton(basePanel, -1, wxString::Format(wxT("%d"),row));
baseSizer->Add(col,1, wxEXPAND);
} else {
wxTextCtrl *txt = new wxTextCtrl(basePanel, -1);
txt->SetMaxLength(10);
baseSizer->Add(txt,1, wxEXPAND);
}
}
}
Center();
}
The output is shown below
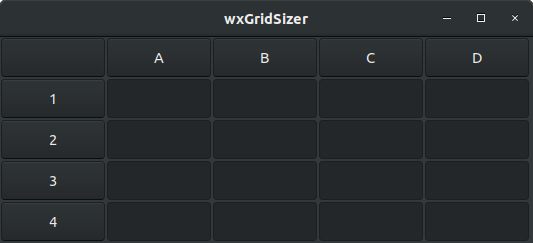
Leave a Reply