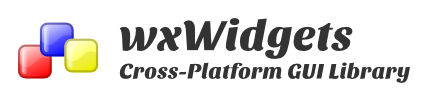
OVERVIEW
The wxBoxSizer sizer is the simplest sizer. It can arrange controls either horizontally or vertically. A wxBoxSizer can be horizontal or vertical. A vertical one would stack the controls vertically and a horizontal one would stack the controls horizontally.
Adding a control to a wxBoxSizer requires the following arguments
- Control or widget to add. This is the only compulsory argument. All other arguments are optional
- Proportion to stretch the control. Default is zero which means the control will not change size if the container is resized. A value of 1 means the control will be resized in proportion to the size of the container. Values higher than 1 mean that the proportion will be x times in comparison to the other sibling controls in the container.
- Flags set anchoring and spacing between controls. The following are valid values and can be used together eg.wxALL | wxALIGN_BOTTOM
- wxLEFT, wxRIGHT, wxTOP, wxBOTTOM, wxALL – which side to set spacing
- wxALIGN_LEFT, wxALIGN_RIGHT, wxALIGN_TOP, wxALIGN_BOTTOM, wxCENTER_VERTICAL, wxCENTER_HORIZONTAL, wxCENTER – alignment of the control
- Spacing value – a numeric value which specifies how much spacing to apply assuming spacing flags have been set in the previous argument
The sample code below creates a window, sets a panel with a vertical wxBoxSizers to it. Three panels with horizontal wxBoxSizers are created and added to the base panel. Each of the three panels define three buttons with various sizing parameters. So what we end up with is three horizontal sized panels within a single vertical sized panel.
SAMPLE CODE
wboxsizer.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class BoxSizer: public wxFrame {
public:
BoxSizer(const wxString &title);
};
wxboxsizer.cpp
#include "wxboxsizer.h"
BoxSizer::BoxSizer(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,200)) {
wxPanel *basePanel = new wxPanel(this, -1);
wxBoxSizer *baseSizer = new wxBoxSizer(wxVERTICAL);
basePanel->SetSizer(baseSizer);
////////////////////////////////////
wxPanel *panel1 = new wxPanel(basePanel, -1);
wxButton *btn1 = new wxButton(panel1, -1, wxT("Button 1"));
wxButton *btn2 = new wxButton(panel1, -1, wxT("Button 2"));
wxButton *btn3 = new wxButton(panel1, -1, wxT("Button 3"));
wxBoxSizer *hbox = new wxBoxSizer(wxHORIZONTAL);
hbox->Add(btn1);
hbox->Add(btn2);
hbox->Add(btn3);
panel1->SetSizer(hbox);
baseSizer->Add(panel1);
////////////////////////////////////////
wxPanel *panel2 = new wxPanel(basePanel, -1);
panel2->SetBackgroundColour(wxColour(0,0,255));
wxButton *btn4 = new wxButton(panel2, -1, wxT("Button 4"));
wxButton *btn5 = new wxButton(panel2, -1, wxT("Button 5"));
wxButton *btn6 = new wxButton(panel2, -1, wxT("Button 6"));
wxBoxSizer *hbox2 = new wxBoxSizer(wxHORIZONTAL);
hbox2->Add(btn4,1);
hbox2->Add(btn5,2, wxEXPAND | wxALL, 10);
hbox2->Add(btn6,4, wxEXPAND );
panel2->SetSizer(hbox2);
baseSizer->Add(panel2);
////////////////////////////////////////
wxPanel *panel3 = new wxPanel(basePanel, -1);
wxButton *btn7 = new wxButton(panel3, -1, wxT("Button 7"));
wxButton *btn8= new wxButton(panel3, -1, wxT("Button 8"));
wxButton *btn9 = new wxButton(panel3, -1, wxT("Button 9"));
wxBoxSizer *hbox3 = new wxBoxSizer(wxHORIZONTAL);
hbox3->Add(btn7,2, wxEXPAND);
hbox3->Add(btn8,2, wxEXPAND);
hbox3->Add(btn9,2, wxEXPAND );
panel3->SetSizer(hbox3);
baseSizer->Add(panel3, 1);
Center();
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "wxboxsizer.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
BoxSizer *app = new BoxSizer(wxT("wxBoxSizer"));
app->Show(true);
}
The output is given below
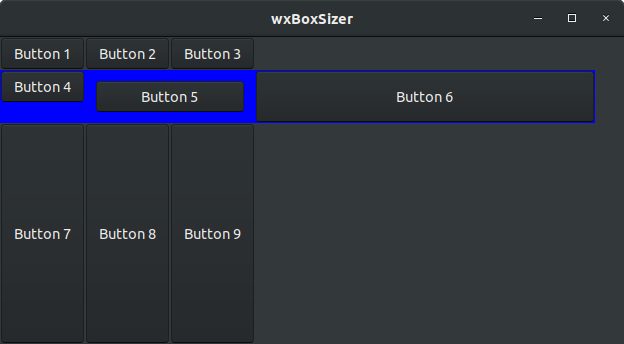
Leave a Reply