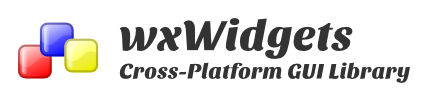
OVERVIEW
Character buffers are used to store buffers in memory for further processing or transferring to other objects. wxWidgets has wxMemoryBuffer which deals with raw bytes, but that is very rarely used. For handing characters, it has two classes:
- wxCharBuffer for handling multi-byte C strings
- wxWCharBuffer for handling wide-char C strings
Most modern applications handle strings as widechar so here we look at how to use wxWCharBuffer. In the sample code below, we initialize a string with printable characters from ASCII code 32 to 126. Then we initialize a wxWCharBuffer to store the contents of the string. The buffer is a copy of the contents of the wxString variable, so when we change the value of wxString, it does not affect the contents of the wxWCharBuffer. Finally we shrink the size of the buffer.
SAMPLE CODE
charbuffer.cpp
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
#include <wx/buffer.h>
int main(int argc, char **argv) {
wxString str = wxT("");
for(int i=32; i< 126; i++) {
char c = (char) i;
str.Append(c);
}
wxPuts(str);
wxWCharBuffer buff(str.Length());
buff = str.c_str();
wxPuts(buff);
wxPuts("------------");
str = wxT("dummy");
wxPuts(str);
wxPuts(buff);
wxPuts("-----------");
buff.shrink(10);
wxPuts(buff);
wxPuts(buff[2]);
}
The output is given below

Leave a Reply