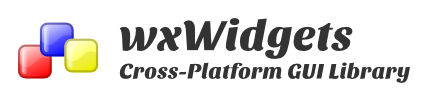
OVERVIEW
Regions are used to change colors in certain areas of the screen by defining different regions and using one of the 4 operations available:
- Union
- Intersect
- Subtract
- Xor
The sample code shows the four operations in action
SAMPLE CODE
regions.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Regions: public wxFrame {
public:
Regions(const wxString &title);
void onPaint(wxPaintEvent &event);
};
regions.cpp
#include "regions.h"
Regions::Regions(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,300)) {
Bind(wxEVT_PAINT, &Regions::onPaint, this);
SetBackgroundColour(wxColour(255,255,255));
}
void Regions::onPaint(wxPaintEvent &event) {
wxPaintDC dc(this);
int x1=50, x2=100,y1=80,y2=150;
int prevx1 = 0, prevx2 = 0, prevy1 = 0, prevy2 = 0;
wxColour *color = new wxColour(40,50,100);
wxColour *rcolor = new wxColour(40,50,200);
wxPenStyle style = wxPENSTYLE_SOLID;
wxRegion *region1, *region2;
for(int i =10; i <= 500; i+=20) {
region1 = new wxRegion(prevx1, prevy1, prevx2, prevy2);
region2 = new wxRegion(x1, y1, x2, y2);
if (i < 100) {
region1->Intersect(*region2);
}
else if (i < 200) {
color = new wxColour(200,145,80);
rcolor = new wxColour(20,145,80);
style = wxPENSTYLE_DOT;
region1->Union(*region2);
} else if (i < 300) {
color = new wxColour(220,45,80);
rcolor = new wxColour(200,105,80);
style = wxPENSTYLE_LONG_DASH;
region1->Xor(*region2);
} else if (i < 400) {
style = wxPENSTYLE_DOT_DASH;
color = new wxColour(220,115,180);
rcolor = new wxColour(220,115,10);
region1->Subtract(*region2);
} else {
color = new wxColour(120,35,18);
rcolor = new wxColour(220,5,180);
style = wxPENSTYLE_SHORT_DASH;
region1->Intersect(*region2);
}
prevy1 = y1; prevx1 = x1; prevy2 = y2; prevx2 = x2;
dc.SetPen(wxPen(*color,1, style));
y1 += 50; x1 += 110;
y2 = y1 + 70; x2 = x1 + 50;
dc.DrawRectangle(x1, y1, x2, y2);
wxRect rrect = region1->GetBox();
dc.SetClippingRegion(rrect);
dc.SetBrush(wxBrush(*rcolor));
dc.DrawRectangle(rrect);
dc.DestroyClippingRegion();
}
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "regions.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
Regions *app = new Regions(wxT("Region"));
app->Show(true);
}
The output is shown below
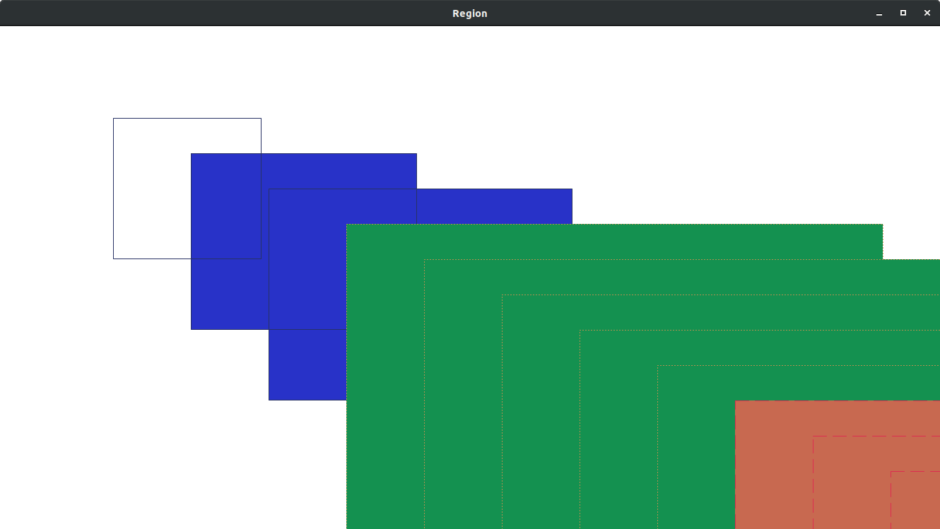
Leave a Reply