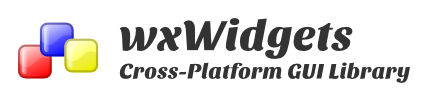
OVERVIEW
All drawing is done by the wxPen object. A pen object can configure color, width and style . Styles can be as shown below
- wxSOLID
- wxDOT
- wxLONG_DASH
- wxSHORT_DASH
- wxDOT_DASH
- wxTRANSPARENT
In the sample code below, we draw rectangles using various parameters with the wxPen object
SAMPLE CODE
pens.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Pens: public wxFrame {
public:
Pens(const wxString &title);
void onPaint(wxPaintEvent &event);
};
pens.cpp
#include "pens.h"
Pens::Pens(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,300)) {
srand(time(NULL));
Bind(wxEVT_PAINT, &Pens::onPaint, this);
SetBackgroundColour(wxColour(255,255,255));
}
void Pens::onPaint(wxPaintEvent &event) {
wxPaintDC dc(this);
int x1=50, x2=100,y1=80,y2=150;
wxColour *color = new wxColour(40,50,100);
wxPenStyle style = wxPENSTYLE_SOLID;
for(int i =10; i <= 500; i+=20) {
if (i < 100) {
// do nothing
}
else if (i < 200) {
color = new wxColour(200,145,80);
style = wxPENSTYLE_DOT;
} else if (i < 300) {
color = new wxColour(220,45,80);
style = wxPENSTYLE_LONG_DASH;
} else if (i < 400) {
style = wxPENSTYLE_DOT_DASH;
color = new wxColour(220,115,180);
} else {
color = new wxColour(120,35,18);
style = wxPENSTYLE_SHORT_DASH;
}
dc.SetPen(wxPen(*color,1, style));
y1 += 10; x1 += 10;
y2 = y1 + 70; x2 = x1 + 50;
dc.DrawRectangle(x1, y1, x2, y2);
}
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "pens.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
Pens *app = new Pens(wxT("Pen"));
app->Show(true);
}
The output is shown below
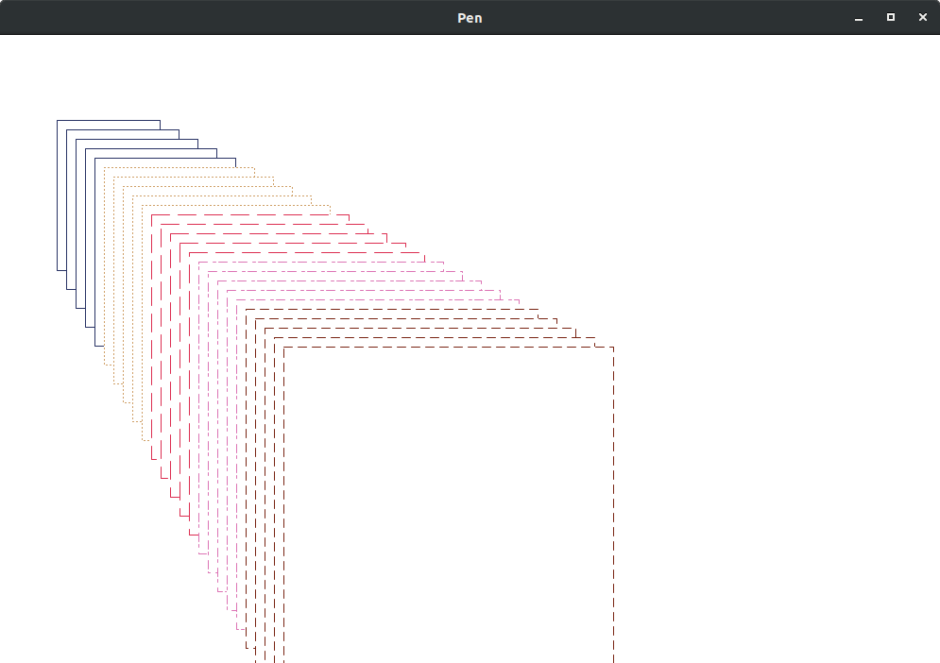
Leave a Reply