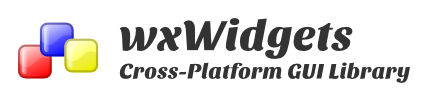
OVERVIEW
DrawPoint() is the simplest GDI function as it draws a point at the given x,y coordinates. In the sample code below, we draw 5000 points at random places in a window.
SAMPLE CODE
points.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Points: public wxFrame {
public:
Points(const wxString &title);
void onPaint(wxPaintEvent &event);
private:
wxPanel *mPanel;
};
points.cpp
#include "points.h"
#include <stdlib.h>
#include <time.h>
Points::Points(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,300)) {
srand(time(NULL));
Bind(wxEVT_PAINT, &Points::onPaint, this);
SetBackgroundColour(wxColour(100,200,10));
}
void Points::onPaint(wxPaintEvent &event) {
wxPaintDC dc(this);
int x=0,y=0;
wxSize size = GetSize();
for(int i =0; i < 5000; i++) {
x = rand() % size.x + 1;
y = rand() % size.y + 1;
dc.DrawPoint(x, y);
}
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "points.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
Points *app = new Points(wxT("Points"));
app->Show(true);
}
The output is given below
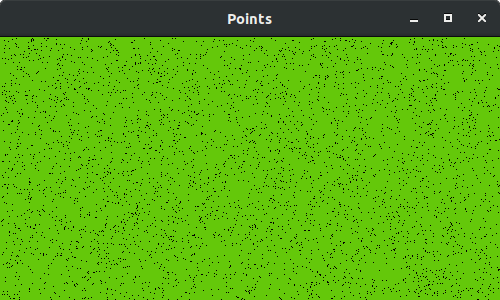
Leave a Reply