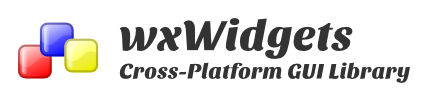
OVERVIEW
We use the DrawLine() method to draw straight lines on the screen. All GDI methods work by overriding the wxEVT_PAINT method. The DrawLine method takes in the starting x.y coordinates and ending x,y coordinates.
SAMPLE CODE
line.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Line: public wxFrame {
public:
Line(const wxString &title);
void onPaint(wxPaintEvent &event);
};
line.cpp
#include "line.h"
Line::Line(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,300)) {
Bind(wxEVT_PAINT, &Line::onPaint, this);
}
void Line::onPaint(wxPaintEvent &event) {
wxPaintDC dc(this);
int x1=5, x2=270,y1=80,y2=0;
for(int i =10; i <= 500; i+=20) {
y2= y1+i;
dc.DrawLine(x1, y1, x2, y2);
}
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "line.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
Line *app = new Line(wxT("Lines"));
app->Show(true);
}
The output is shown below
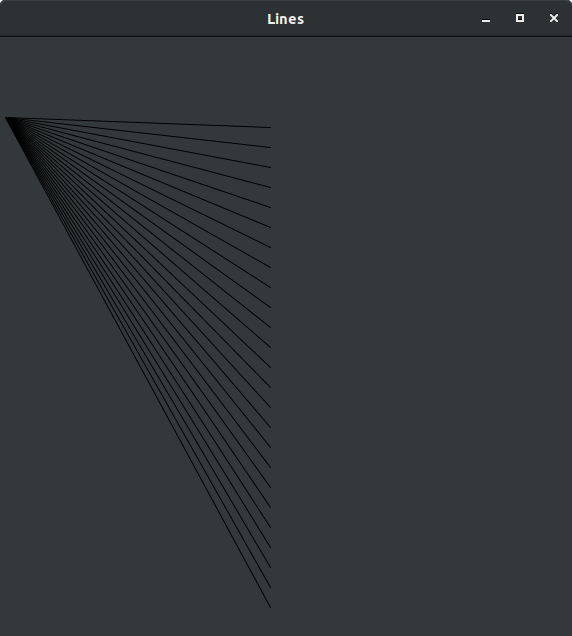
Leave a Reply