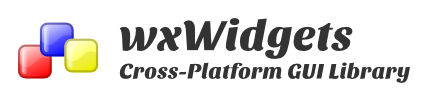
OVERVIEW
A wxListBox is a list which displays strings as items. It can select either a single item or multiple items. In the code below, we start with an empty wxListBox and then use buttons to add/edit/delete items .
SAMPLE CODE
wxlistbox.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class ListBox: public wxFrame {
public:
ListBox(const wxString &title);
void onAdd(wxCommandEvent &event);
void onEdit(wxCommandEvent &event);
void onDelete(wxCommandEvent &event);
void onClear(wxCommandEvent &event);
private:
wxListBox *mList;
};
wxlistbox.cpp
#include "wxlistbox.h"
ListBox::ListBox(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,250)) {
wxPanel *basePanel = new wxPanel(this,-1);
wxBoxSizer *hbox = new wxBoxSizer(wxHORIZONTAL);
basePanel->SetSizer(hbox);
wxPanel *leftPanel = new wxPanel(basePanel, -1);
wxBoxSizer *vbox1 = new wxBoxSizer(wxVERTICAL);
leftPanel->SetSizer(vbox1);
wxPanel *rightPanel = new wxPanel(basePanel, -1);
wxBoxSizer *vbox2 = new wxBoxSizer(wxVERTICAL);
rightPanel->SetSizer(vbox2);
mList = new wxListBox(leftPanel,-1, wxDefaultPosition, wxDefaultSize);
vbox1->Add(mList, 1, wxEXPAND);
wxButton *btnAdd = new wxButton(rightPanel, -1, wxT("Add"));
wxButton *btnEdit = new wxButton(rightPanel, -1, wxT("Edit"));
wxButton *btnDelete = new wxButton(rightPanel, -1, wxT("Delete"));
wxButton *btnClear = new wxButton(rightPanel, -1, wxT("Clear"));
btnAdd->Bind(wxEVT_BUTTON, &ListBox::onAdd, this);
btnEdit->Bind(wxEVT_BUTTON, &ListBox::onEdit, this);
btnDelete->Bind(wxEVT_BUTTON, &ListBox::onDelete, this);
btnClear->Bind(wxEVT_BUTTON, &ListBox::onClear, this);
vbox2->Add(btnAdd, 1, wxEXPAND | wxALL, 5);
vbox2->Add(btnEdit, 1, wxEXPAND | wxALL, 5);
vbox2->Add(btnDelete, 1, wxEXPAND | wxALL, 5);
vbox2->Add(btnClear, 1, wxEXPAND | wxALL, 5);
hbox->Add(leftPanel, 2, wxEXPAND | wxALL, 5);
hbox->Add(rightPanel, 1, wxEXPAND | wxALL, 5);
Center();
}
void ListBox::onAdd(wxCommandEvent &event) {
wxString string = wxGetTextFromUser(wxT("Add new Item"));
if (string != "") {
mList->Append(string);
}
}
void ListBox::onEdit(wxCommandEvent &event) {
int selected = mList->GetSelection();
if (selected == -1) {
wxMessageDialog *dlg = new wxMessageDialog(NULL, wxT("No item has been selected in the listbox"), wxT(""));
dlg->ShowModal();
return;
}
wxString selString = mList->GetString(selected);
wxString changed = wxGetTextFromUser(wxT("Edit"), wxT(""), selString);
if (changed != "") {
mList->Delete(selected);
mList->Insert(changed, selected);
}
}
void ListBox::onDelete(wxCommandEvent &event) {
int selected = mList->GetSelection();
if (selected == -1) {
wxMessageDialog *dlg = new wxMessageDialog(NULL, wxT("No item has been selected in the listbox"), wxT(""));
dlg->ShowModal();
return;
}
mList->Delete(selected);
}
void ListBox::onClear(wxCommandEvent &event) {
wxMessageDialog* dial = new wxMessageDialog(NULL, wxT("Are you sure?"), wxT(""),
wxYES_NO | wxNO_DEFAULT | wxICON_QUESTION);
int retVal = dial->ShowModal();
if (retVal == wxID_YES) {
mList->Clear();
}
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "wxlistbox.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
ListBox *app = new ListBox(wxT("ListBox"));
app->Show(true);
}
The output is shown below
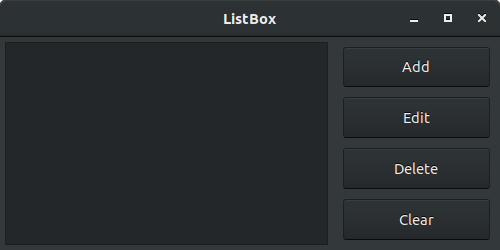
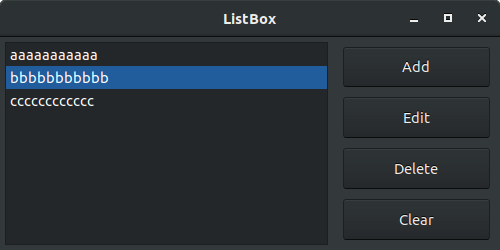
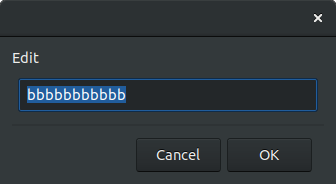
Leave a Reply