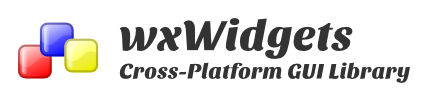
OVERVIEW
We have used wxStaticText a lot in the previous examples. It is used to display either a single or multiple lines of text. In the sample code below, we will display multiple lines of text in a panel.
SAMPLE CODE
statictext.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class StaticText: public wxFrame {
public:
StaticText(const wxString &title);
};
statictext.cpp
#include "statictext.h"
StaticText::StaticText(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,200)) {
wxPanel *panel = new wxPanel(this, -1);
panel->SetBackgroundColour(wxColour(200,200,100));
wxStaticText *label = new wxStaticText(panel, -1, wxT(""), wxPoint(20,10), wxDefaultSize);
wxString text = wxT("This is line 1.\nThis is line 2.\nThis is line 3.\n");
label->SetLabel(text);
Center();
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "statictext.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
StaticText *app = new StaticText(wxT("StaticText"));
app->Show(true);
}
The output is shown below
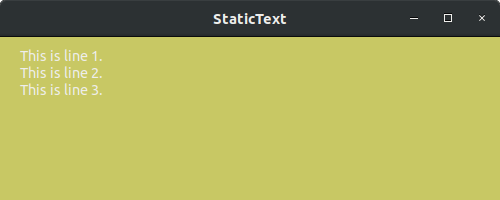
Leave a Reply