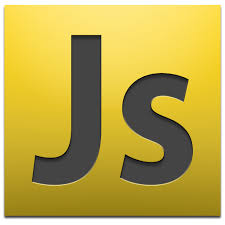
OVERVIEW
There are some small but important differences between using var, let and const:
A var can be re-declared and reassigned different values. Let variables can be reassigned different values but cannot be redeclared. A const can only be declared and assigned once.
In terms of scope a var variable is either function scoped or global scoped. That means if a var is declared within a function, then it is visible throughout the function and if a var is declared at the global level then it is visible everywhere. If a var is declared at the global and also at the function level then the function level declaration overrides the global declaration.
Let variables and const variable have block scope. A block scope is any block of code within opening and closing curly braces – {}. So they have much more fine scope control.
The sample code below shows the various characteristics describe above
SAMPLE CODE
<html>
<head>
<script>
var varItem = "one";
document.write(varItem + "<br>");
var varItem = 24.55;
document.write(varItem + "<br>");
document.write("<hr>");
let letItem = "two";
document.write(letItem + "<br>");
//let letItem = 189; // this line will give an error
document.write(letItem + "<br>");
document.write("<hr>");
const constItem =449;
document.write(constItem + "<br>");
//constItem = 444; // this line will give an error
document.write(constItem + "<br>");
let globalLet = "global";
document.write("globalLet in global scope=" + globalLet + "<br>");
func();
var testVar = "test1";
function func() {
var testVar = "test2";
if (1 < 2) {
var funcVar = "functionVar";
}
let globalLet = "function";
document.write("globalLet in function scope=" + globalLet + "<br>");
document.write("testar=" + testVar + "<br>");
document.write("funcVar=" + funcVar);
}
</script>
</head>
<body>
</body>
</html>
The output is shown below
one 24.55 two two 449 449 globalLet in global scope=global globalLet in function scope=function testar=test2 funcVar=functionVar
Leave a Reply