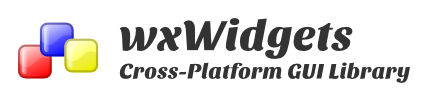
OVERVIEW
wxWidgets provides support for reading and writing the following archive formats: zip, tar and zlib. Of these, the zip format is the most commonly used archive, so the sample code below shows how to write and read zip files.
The sample code creates a zip file which contains files from the current working directory based on a wildcard filter. In this case it looks for all files which have the extension of .cpp. You can change it to anything. Once the zip is created, the second button reads the zip files and lists the file names and their size in bytes.
SAMPLE CODE
archive.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Archive: public wxFrame {
public:
Archive(const wxString &title);
private:
void onZip(wxCommandEvent &event);
void onUnzip(wxCommandEvent &event);
wxTextCtrl *mText;
};
archive.cpp
#include "archive.h"
#include <wx/wfstream.h>
#include <wx/zipstrm.h>
#include <wx/archive.h>
#include <wx/dir.h>
Archive::Archive(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(600,400)) {
wxPanel *panel = new wxPanel(this, -1);
wxBoxSizer *vbox = new wxBoxSizer(wxVERTICAL);
panel->SetSizer(vbox);
mText = new wxTextCtrl(panel, -1, wxT(""), wxDefaultPosition, wxDefaultSize,
wxTE_MULTILINE | wxTE_READONLY);
wxButton *btnZip = new wxButton(panel, -1, wxT("Create Zip"));
wxButton *btnUnzip = new wxButton(panel, -1, wxT("List Zip Contents"));
vbox->Add(btnZip,1, wxEXPAND | wxALL, 4);
vbox->Add(btnUnzip,1, wxEXPAND | wxALL, 4);
vbox->Add(mText,4,wxEXPAND | wxALL,2);
btnZip->Bind(wxEVT_BUTTON, &Archive::onZip, this);
btnUnzip->Bind(wxEVT_BUTTON, &Archive::onUnzip, this);
Center();
}
void Archive::onZip(wxCommandEvent &event) {
wxString msg = wxT("");
mText->Clear();
msg.Append(wxT("Creating test.zip.\n"));
wxFFileOutputStream *fos = new wxFFileOutputStream(wxT("test.zip"));
wxZipOutputStream *zos = new wxZipOutputStream(fos);
wxDir dir(wxGetCwd());
if (!dir.IsOpened()) {
msg.Append(wxT("Error opening current dir"));
} else {
wxString fileName;
bool retVal = dir.GetFirst(&fileName, wxT("*.cpp"));
while (retVal) {
zos->PutNextEntry(fileName);
wxFFileInputStream *fis = new wxFFileInputStream(fileName);
zos->Write(*fis);
msg.Append("Added ").Append(fileName).Append(wxT("\n"));
retVal = dir.GetNext(&fileName);
}
}
zos->Close();
fos->Close();
msg.Append(wxT("Closed zip file\n"));
mText->AppendText(msg);
}
void Archive::onUnzip(wxCommandEvent &event) {
wxString msg = wxT("");
mText->Clear();
if (wxFileExists(wxT("test.zip"))) {
wxFFileInputStream *fis = new wxFFileInputStream(wxT("test.zip"));
wxZipInputStream *zis = new wxZipInputStream(*fis);
wxArchiveEntry *entry = zis->GetNextEntry();
while (entry != NULL) {
off_t size = entry->GetSize();
msg.Append(entry->GetName()).Append(wxT(" ("))
.Append(wxString::Format(wxT("%lu"), size))
.Append(wxT(" bytes)"))
.Append(wxT("\n"));
entry = zis->GetNextEntry();
}
mText->AppendText(msg);
} else {
msg.Append(wxT("test.zip does not exist"));
}
mText->AppendText(msg);
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "archive.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
Archive *app = new Archive(wxT("Archive Handling"));
app->Show(true);
}
The output is shown below
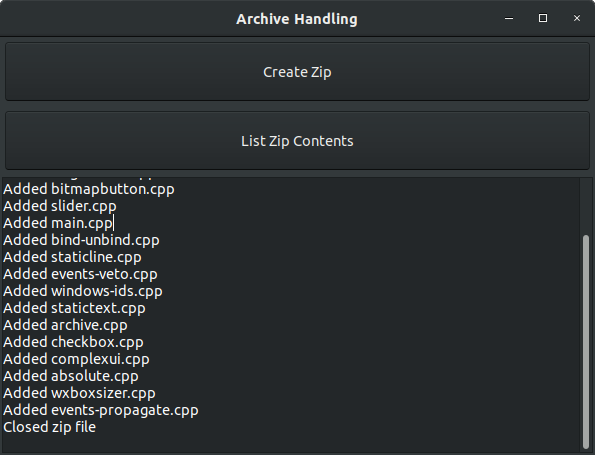
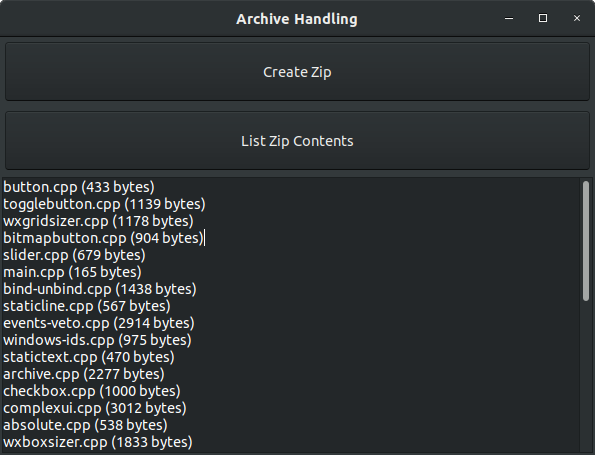
Leave a Reply