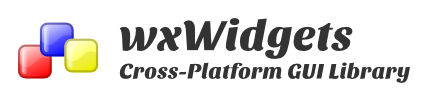
OVERVIEW
Drawing text is similar to drawing lines, except here we pass a string as an argument along with the x,y coordinates of where it has to be shown
SAMPLE CODE
drawtext.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class DrawText: public wxFrame {
public:
DrawText(const wxString &title);
void onPaint(wxPaintEvent &event);
};
drawtext.cpp
#include "drawtext.h"
DrawText::DrawText(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,300)) {
Bind(wxEVT_PAINT, &DrawText::onPaint, this);
}
void DrawText::onPaint(wxPaintEvent &event) {
wxPaintDC dc(this);
int x=80,y=80;
for(int i =10; i <= 500; i+=10) {
dc.DrawText(wxT("This is a line of text"), x, y);
y += i; x+= i;
}
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "drawtext.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
DrawText *app = new DrawText(wxT("DrawText"));
app->Show(true);
}
The output is shown below
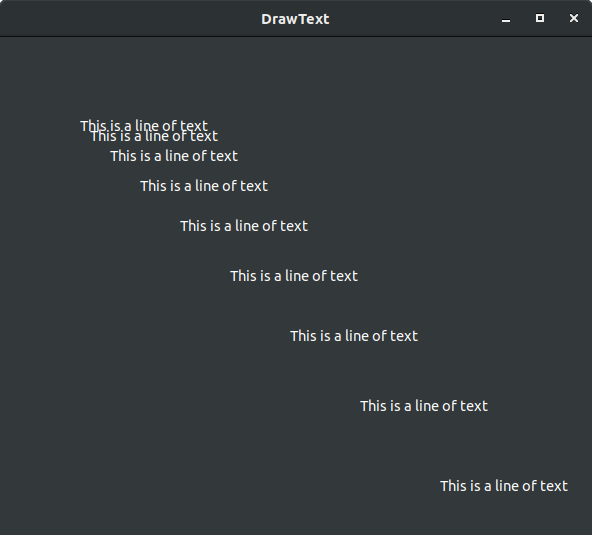
Leave a Reply