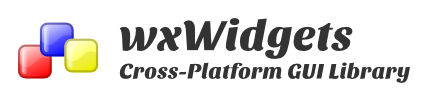
OVERVIEW
wxGrid sets up a grid with rows and columns, much like a spreadsheet and then add text to each of the cells. That is the basic wxGrid implementation. You can subclass it to create more sophisticated tabular data display of complex objects.
The sample code below creates a wxGrid with custom colors, content and cell formatting. The first column is read-only containing employee codes. The second column allows entry of any string for employee name. The third column only accepts float or double values for salary amount.
SAMPLE CODE
grid.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Grid: public wxFrame {
public:
Grid(const wxString &title);
private:
};
grid.cpp
#include "grid.h"
#include <wx/grid.h>
Grid::Grid(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(700,550)) {
wxPanel *panel = new wxPanel(this, -1);
wxBoxSizer *vbox = new wxBoxSizer(wxVERTICAL);
panel->SetSizer(vbox);
wxGrid *grid = new wxGrid(panel, -1);
grid->CreateGrid(40,3);
grid->SetColSize(0,100);
grid->SetColSize(1,400);
grid->SetColSize(2,100);
grid->SetColFormatFloat(2,6,2);
wxString empCode = wxT("Emp#");
for(int i = 0; i < 40; i++) {
empCode = wxT("Emp#");
grid->SetCellValue(i, 0, empCode.Append(wxString::Format(wxT("%d"), (i+1))));
grid->SetReadOnly(i,0);
grid->SetCellTextColour(i, 0, *wxGREEN);
grid->SetCellBackgroundColour(i, 0, wxColor(100,100,100));
grid->SetCellBackgroundColour(i, 1, wxColor(200,80,45));
grid->SetCellBackgroundColour(i, 2, wxColor(45,19,177));
}
vbox->Add(grid, 1, wxEXPAND);
Center();
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "grid.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
Grid *app = new Grid(wxT("Grid"));
app->Show(true);
}
The output is shown below
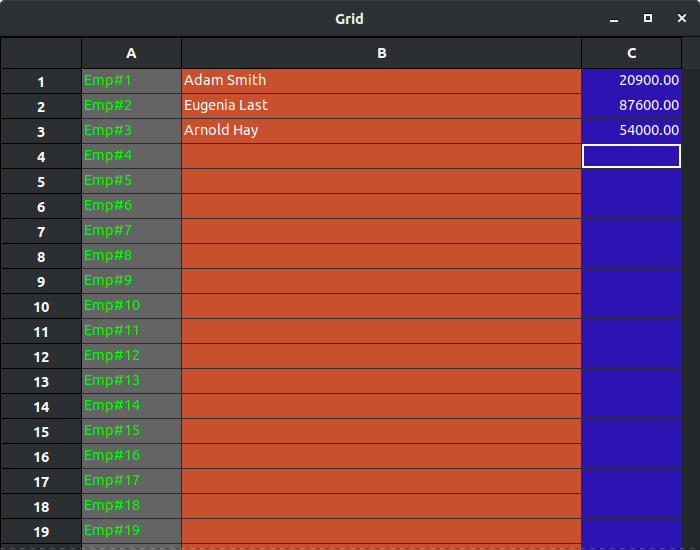
Leave a Reply