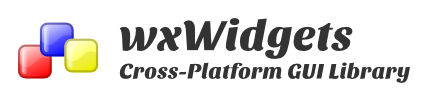
OVERVIEW
In the sample code below, we use the wxFileDialog to select a text file , whose contents are then shown in a textbox.
SAMPLE CODE
dialog-file.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class DialogFile: public wxFrame {
public:
DialogFile(const wxString& title);
void OnClick(wxCommandEvent &event);
private:
wxTextCtrl *mTxt;
};
dialog-file.cpp
#include "dialog-file.h"
DialogFile::DialogFile(const wxString& title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,400)) {
wxPanel *panelMain = new wxPanel(this, -1);
wxBoxSizer *vbox = new wxBoxSizer(wxVERTICAL);
panelMain->SetSizer(vbox);
wxButton *btn1 = new wxButton(panelMain, -1, wxT("Choose Text File"));
mTxt = new wxTextCtrl(panelMain, -1, wxT(""), wxPoint(-1,-1), wxSize(-1,-1), wxTE_MULTILINE);
vbox->Add(btn1, 0, wxALL | wxCENTER,5);
vbox->Add(mTxt, 1, wxALL | wxEXPAND | wxCENTER, 5);
btn1->Bind(wxEVT_BUTTON, &DialogFile::OnClick, this);
Center();
}
void DialogFile::OnClick(wxCommandEvent &event) {
wxFileDialog *dlg = new wxFileDialog(this, wxT("Open Text File"), wxT(""), wxT(""), wxT("txt files (*.txt)|*.txt"),
wxFD_OPEN | wxFD_FILE_MUST_EXIST);
int retVal = dlg->ShowModal();
if (retVal == wxID_OK) {
wxString path = dlg->GetPath();
mTxt->LoadFile(path);
}
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "dialog-file.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
DialogFile *app = new DialogFile(wxT("File Open Dialog "));
app->Show(true);
}
The output is shown below
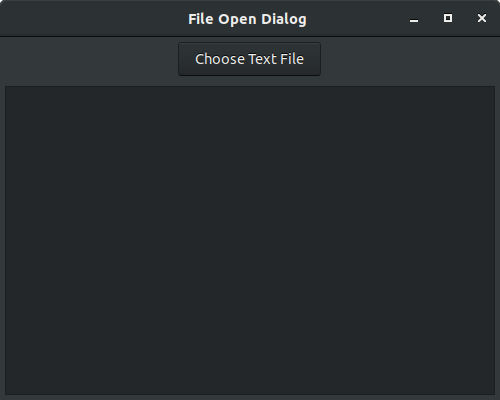
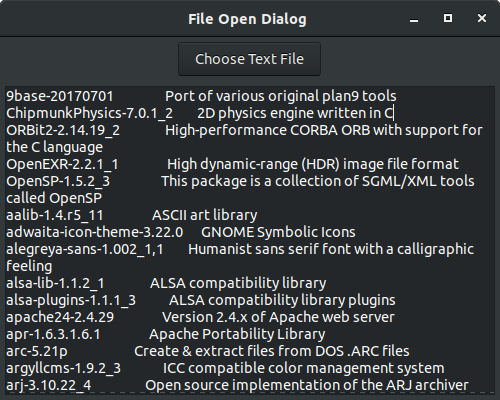
Leave a Reply