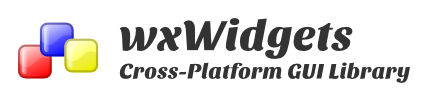
OVERVIEW
As mentioned before in a previous section, Bind is the preferred way to do event handling. The main advantage of Bind is that you can bind events at runtime. We look at an example of this in the code below. We use Bind and Unbind to attach 3 different event handlers to a single button , one at a time.
We use an inbuilt function called CallAfter, which we will explore in later sections. The basic purpose of CallAfter is to execute a method when wxWidgets is idle. The reason why we dont call the RemoveHandler directly is because it is not a good idea to remove an event handler from a widget while it is still executing the event handler. Using CallAfter makes sure the event is unbound after it has finished executing.
SAMPLE CODE
bind-unbind.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class BindUnbind: public wxFrame {
public:
BindUnbind(const wxString &title);
void OnClick1(wxCommandEvent &event);
void OnClick2(wxCommandEvent &event);
void OnClick3(wxCommandEvent &event);
void RemoveHandler(int remove, int add);
private:
wxButton *btn1;
};
bind-unbind.cpp
#include "bind-unbind.h"
BindUnbind::BindUnbind(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,200)) {
wxPanel *panel = new wxPanel(this, -1);
wxBoxSizer *hbox = new wxBoxSizer(wxHORIZONTAL);
panel->SetSizer(hbox);
btn1 = new wxButton(panel, -1, wxT("click here"));
hbox->Add(btn1, 1, wxEXPAND | wxALL, 35);
btn1->Bind(wxEVT_BUTTON, &BindUnbind::OnClick1, this);
Center();
}
void BindUnbind::OnClick1(wxCommandEvent &event) {
btn1->SetLabel(wxT("in OnClick1"));
CallAfter(&BindUnbind::RemoveHandler, 1, 2);
}
void BindUnbind::OnClick2(wxCommandEvent &event) {
btn1->SetLabel(wxT("in OnClick2"));
CallAfter(&BindUnbind::RemoveHandler, 2, 3);
}
void BindUnbind::OnClick3(wxCommandEvent &event) {
btn1->SetLabel(wxT("in OnClick3"));
CallAfter(&BindUnbind::RemoveHandler, 3, 1);
}
void BindUnbind::RemoveHandler(int remove, int add) {
if (remove == 1)
btn1->Unbind(wxEVT_BUTTON, &BindUnbind::OnClick1, this);
else if (remove == 2)
btn1->Unbind(wxEVT_BUTTON, &BindUnbind::OnClick2, this);
else if (remove == 3)
btn1->Unbind(wxEVT_BUTTON, &BindUnbind::OnClick3, this);
if (add == 1)
btn1->Bind(wxEVT_BUTTON, &BindUnbind::OnClick1, this);
else if (add == 2)
btn1->Bind(wxEVT_BUTTON, &BindUnbind::OnClick2, this);
else if (add == 3)
btn1->Bind(wxEVT_BUTTON, &BindUnbind::OnClick3, this);
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "bind-unbind.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
BindUnbind *app = new BindUnbind(wxT("Bind Unbind"));
app->Show(true);
}
The output is shown below
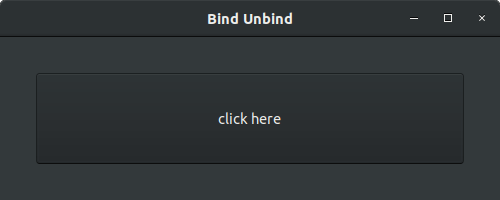
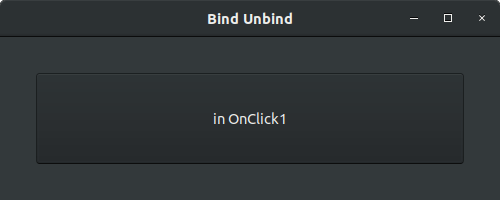
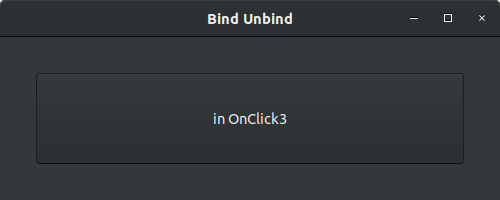
Leave a Reply