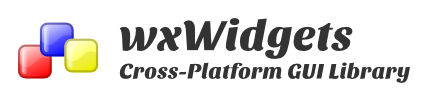
OVERVIEW
Continuing from the previous section where we looked at event propagation, in this section we see how to cancel processing events. Event processing may be cancelled by either the Veto() method or Skip(false) method. Events derived from wxCommandEvent do not have a Veto method. They use the Skip method. Events which are not derived from wxCommandEvent have a Veto method(). If you dont specify the false argument in Skip() then the event is propagated.
The sample code below uses Skip to veto command events and Veto to cancel a wxCloseEvent.
SAMPLE CODE
events-veto.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class EventsVeto: public wxFrame {
public:
EventsVeto(const wxString &title);
void OnClose(wxCloseEvent &event);
void OnClick(wxCommandEvent &event);
void OnClick2(wxCommandEvent &event);
void OnClick3(wxCommandEvent &event);
void OnClick4(wxCommandEvent &event);
};
const int ID_PANEL_1 = 101;
const int ID_PANEL_1_1 = 102;
const int ID_BUTTON_1 = 200;
events-veto.cpp
#include "events-veto.h"
EventsVeto::EventsVeto(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,200)) {
this->Bind(wxEVT_CLOSE_WINDOW, &EventsVeto::OnClose, this);
wxPanel *basePanel = new wxPanel(this, -1);
wxBoxSizer *baseSizer = new wxBoxSizer(wxVERTICAL);
basePanel->SetSizer(baseSizer);
wxPanel *panel1 = new wxPanel(basePanel, ID_PANEL_1);
panel1->SetBackgroundColour(wxColour(100,200,100));
wxBoxSizer *vbox = new wxBoxSizer(wxVERTICAL);
panel1->SetSizer(vbox);
wxPanel *panel11 = new wxPanel(panel1, ID_PANEL_1_1);
panel11->SetBackgroundColour(wxColour(50,180,45));
wxBoxSizer *hbox = new wxBoxSizer(wxHORIZONTAL);
panel11->SetSizer(hbox);
wxButton *btn = new wxButton(panel11, ID_BUTTON_1, wxT("Click this button"));
hbox->Add(btn, 0, wxALIGN_CENTER);
vbox->Add(panel11, 1, wxALL | wxEXPAND, 50);
baseSizer->Add(panel1, 1, wxALL | wxEXPAND,5);
btn->Bind(wxEVT_BUTTON, &EventsVeto::OnClick, this);
panel11->Bind(wxEVT_COMMAND_BUTTON_CLICKED, &EventsVeto::OnClick2, this);
panel1->Bind(wxEVT_COMMAND_BUTTON_CLICKED, &EventsVeto::OnClick3, this);
this->Bind(wxEVT_COMMAND_BUTTON_CLICKED, &EventsVeto::OnClick4, this);
Center();
}
void EventsVeto::OnClose(wxCloseEvent &event) {
wxMessageDialog *dlg = new wxMessageDialog(NULL,
wxT("Are you sure you want to close?"), wxT("Question"), wxYES_NO);
int retVal = dlg->ShowModal();
dlg->Destroy();
if (retVal == wxID_NO) {
event.Veto();
return;
}
Destroy();
}
void EventsVeto::OnClick(wxCommandEvent &event) {
wxMessageDialog *dlg = new wxMessageDialog(NULL,
wxT("Veto at the button level?"), wxT("Question"), wxYES_NO);
int retVal = dlg->ShowModal();
dlg->Destroy();
if (retVal == wxID_YES) {
event.Skip(false);
return;
}
wxString msg= "Click detected in btn ";
wxMessageBox(msg);
event.Skip();
}
void EventsVeto::OnClick2(wxCommandEvent &event) {
wxMessageDialog *dlg = new wxMessageDialog(NULL,
wxT("Veto at the panel11 level?"), wxT("Question"), wxYES_NO);
int retVal = dlg->ShowModal();
dlg->Destroy();
if (retVal == wxID_YES) {
event.Skip(false);
return;
}
wxString msg= "Click detected in panel11 ";
wxMessageBox(msg);
event.Skip();
}
void EventsVeto::OnClick3(wxCommandEvent &event) {
wxMessageDialog *dlg = new wxMessageDialog(NULL,
wxT("Veto at the panel1 level?"), wxT("Question"), wxYES_NO);
int retVal = dlg->ShowModal();
dlg->Destroy();
if (retVal == wxID_YES) {
event.Skip(false);
return;
}
wxString msg= "Click detected in panel1";
wxMessageBox(msg);
event.Skip();
}
void EventsVeto::OnClick4(wxCommandEvent &event) {
wxString msg= "Click detected in window frame ";
wxMessageBox(msg);
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "events-veto.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
EventsVeto *app = new EventsVeto(wxT("Events Veto"));
app->Show(true);
}
The output is shown below
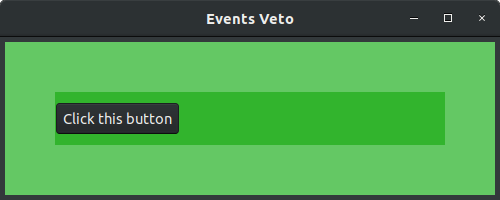
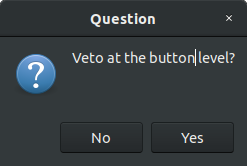
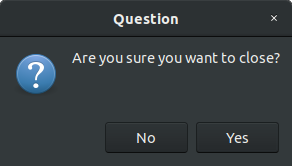
Leave a Reply