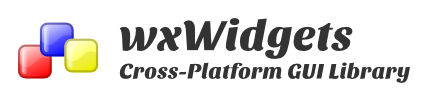
OVERVIEW
We setup a simple GUI in this section and use an event table to handle various events. The event table handles the clicks on three buttons and the text change event in the textbox. We have used specific IDs to identify each control since its used to connect or identify the source object in the event handler.
SAMPLE CODE
events-table.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class EventsTable: public wxFrame {
public:
EventsTable(const wxString& title);
void OnClick(wxCommandEvent &event);
void OnText(wxCommandEvent &event);
private:
wxStaticText *m_lbl;
wxTextCtrl *m_edit;
DECLARE_EVENT_TABLE()
};
const int ID_BTN_1 = 101;
const int ID_BTN_2 = 102;
const int ID_BTN_3 = 103;
const int ID_TXT_1 = 104;
events-table.cpp
#include "events-table.h"
EventsTable::EventsTable(const wxString& title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,200)) {
wxPanel *panelMain = new wxPanel(this, -1);
wxBoxSizer *vbox = new wxBoxSizer(wxVERTICAL);
panelMain->SetSizer(vbox);
wxPanel *panelBase = new wxPanel(panelMain, -1);
wxBoxSizer *hbox = new wxBoxSizer(wxHORIZONTAL);
panelBase->SetSizer(hbox);
wxButton *btn1 = new wxButton(panelBase, ID_BTN_1, wxT("Button 1"));
wxButton *btn2 = new wxButton(panelBase, ID_BTN_2, wxT("Button 2"));
wxButton *btn3 = new wxButton(panelBase, ID_BTN_3, wxT("Button 3"));
m_edit = new wxTextCtrl(panelBase, ID_TXT_1, wxT(""));
hbox->Add(btn1, 1, wxALL,5);
hbox->Add(btn2, 1, wxALL,5);
hbox->Add(btn3, 1, wxALL,5);
hbox->Add(m_edit, 2, wxALL,5);
vbox->Add(panelBase,1, wxALL,2);
m_lbl = new wxStaticText(panelMain, -1, wxT("show event handling here"));
vbox->Add(m_lbl, 1, wxALL | wxALIGN_CENTER);
Center();
}
BEGIN_EVENT_TABLE(EventsTable, wxFrame)
EVT_BUTTON(ID_BTN_1, EventsTable::OnClick)
EVT_BUTTON(ID_BTN_2, EventsTable::OnClick)
EVT_BUTTON(ID_BTN_3, EventsTable::OnClick)
EVT_TEXT(ID_TXT_1,EventsTable::OnText)
END_EVENT_TABLE()
void EventsTable::OnClick(wxCommandEvent &event) {
wxString strId = wxString::Format(wxT("%d"), event.GetId());
strId.Append(wxString(" clicked"));
m_lbl->SetLabel(strId);
}
void EventsTable::OnText(wxCommandEvent &event) {
m_lbl->SetLabel(m_edit->GetValue());
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "events-table.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
EventsTable *app = new EventsTable(wxT("Events Table"));
app->Show(true);
}
The output is shown below
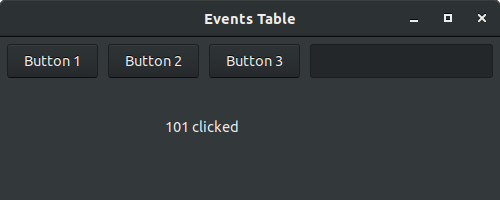
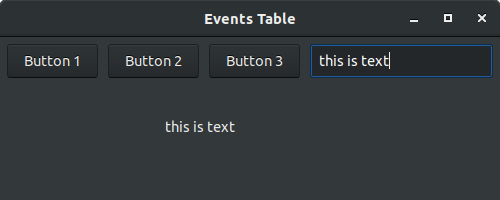
Leave a Reply