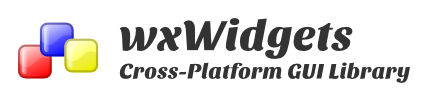
OVERVIEW
We take a first look at handling events in wxWidgets. The simplest event is a button click. There are 3 ways of handling events:
- Using an Event Table
- Using Connect()
- Using Bind()
The first two methods are not recommended anymore and wxWidgets encourages using Bind() for all event handling as its simpler and safer . So all sample code in this series, will only use Bind().
The sample code below, creates two buttons in two panels. Clicking on them will change the parent panel background color to red.
SAMPLE CODE
events.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class TestEvents: public wxFrame {
public:
TestEvents(const wxString& title);
void leftBtnClick(wxCommandEvent &evt);
void rightBtnClick(wxCommandEvent &evt);
wxPanel *panLeft;
wxPanel *panRight;
};
events.cpp
#include "events.h"
TestEvents::TestEvents(const wxString& title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,200)) {
panLeft = new wxPanel(this, -1, wxPoint(0,0), wxSize(250,100));
panRight = new wxPanel(this, -1, wxPoint(251,0), wxSize(250,100));
panLeft->SetBackgroundColour(wxColour(100,200,200));
panRight->SetBackgroundColour(wxColour(100,100,100));
wxButton *btn1 = new wxButton(panLeft,-1, wxT("Left Panel"), wxPoint(5,5));
wxButton *btn2 = new wxButton(panRight,-1, wxT("Right Panel"), wxPoint(5,5));
btn1->Bind(wxEVT_BUTTON, &TestEvents::leftBtnClick, this);
btn2->Bind(wxEVT_BUTTON, &TestEvents::rightBtnClick, this);
SetMinSize(GetSize());
SetMaxSize(GetSize());
Center();
}
void TestEvents::leftBtnClick(wxCommandEvent &evt) {
panLeft->SetBackgroundColour(wxColour(255,0,0));
}
void TestEvents::rightBtnClick(wxCommandEvent &evt) {
panRight->SetBackgroundColour(wxColour(255,0,0));
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "events.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
TestEvents *app = new TestEvents(wxT("Test Events"));
app->Show(true);
}
The output is shown below
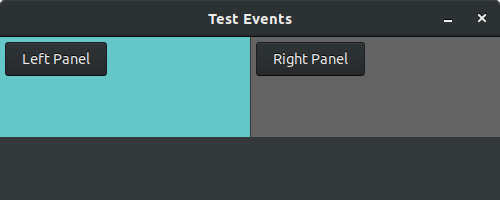
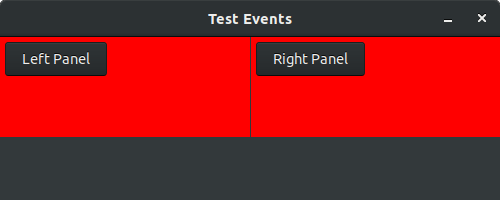
Leave a Reply