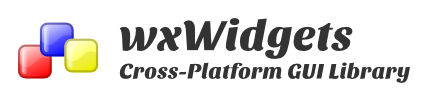
OVERVIEW
Panels are the basic containers which are used while creating UI layouts. They are created within the main wxFrame and help create top-level grouping and positioning of controls. If layouts are not being used, then all positioning is done using fixed (x,y) points. We are not covering layouts here so we are hard-coding the placement of all controls. Since hard-coded placements are not responsive to change in window size, we have made the wxFrame non-resizable in the code below.
SAMPLE CODE
panels.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Panels: public wxFrame {
public:
Panels(const wxString& title);
};
panels.cpp
#include "panels.h"
Panels::Panels(const wxString& title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,200)) {
wxPanel *panLeft = new wxPanel(this, -1, wxPoint(0,0), wxSize(250,100));
wxPanel *panRight = new wxPanel(this, -1, wxPoint(251,0), wxSize(250,100));
panLeft->SetBackgroundColour(wxColour(100,200,200));
panRight->SetBackgroundColour(wxColour(100,100,100));
wxStaticText *label1 = new wxStaticText(panLeft,-1, wxT("Left Panel"), wxPoint(5,5));
wxStaticText *label2 = new wxStaticText(panRight,-1, wxT("Right Panel"), wxPoint(5,5));
SetMinSize(GetSize());
SetMaxSize(GetSize());
Center();
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "panels.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
Panels *app = new Panels(wxT("Panels"));
app->Show(true);
}
The output is shown below
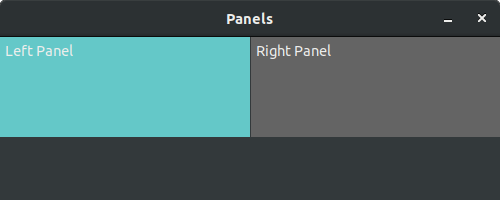
Leave a Reply