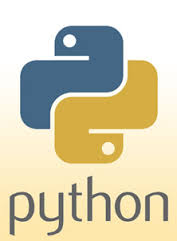
Now that we have finished looking at data types, lets look at writing logic in Python. A control structure is a language construct which controls the execution of code. Without control structures , python would execute statements from the first to the last statement one by one. Control structures enable us to divert that execution into different paths.
There are two kinds of control structures:
- iteration
- selection
Iteration
An iteration is a control structure which repeats statements a certain number of times. Iteration consists of two statements:
while
The while statement will execute statements within it as long as a condition returns True. Some examples are shown below:
>>> >>> >>> i = 10; >>> while i <= 100: ... print "i=%d" %i ... i = i + 10 ... i=10 i=20 i=30 i=40 i=50 i=60 i=70 i=80 i=90 i=100 >>>
>>> end = False >>> while end <> True: ... enter = input("Shall I stop? ") ... if enter == "yes": ... end = True ... Shall I stop? "no" Shall I stop? "maybe" Shall I stop? "wait for a while" Shall I stop? "yes" >>>
While stops execution when a condition becomes True. This can be used for a lot of complex code where multiple conditions need to be checked.
for
The for control structure is simpler than the while statement since it iterates for a fixed number of times in sequence. It does not work with logical conditions. It works on a range of values.
>>> >>> for i in range(0,10): ... print i ... 0 1 2 3 4 5 6 7 8 9
>>> list = ["a", "b", "d", "e"] >>> for item in list: ... print item ... a b d e >>>
The advantage of for over while is that it is faster in execution because the iteration is fixed and the python compiler knows when the iteration will end. The drawback of for loop is that it can only work on a range of values. and while is more suited for complex iterations.
Selection
Selection enables the code to make a decision about which execution path to take based on a logical condition. We use the if statement
>>> >>> age = 25 >>> if age < 20: ... print "You are too young" ... You are too old >>>
If can be used with else
>>> >>> >>> age = 10 >>> if age < 20: ... print "less than 20" ... else: ... print "more than 20" ... less than 20 >>>
If can be used with multiple elif (short for else if)
>>> >>> type = "vegetarian" >>> if type == "vegetarian": ... print "veg" ... elif type == "non-vegetarian": ... print "non-veg" ... else: ... print "what on earth are you" ... veg >>>
Here is an example which uses both while and if
>>> counter = 0 >>> while counter <= 20: ... if counter % 2 == 0: ... print "%d is divisible by 2" % counter ... if counter % 3 == 0: ... print "%d is divisible by 3" % counter ... if counter % 4 == 0: ... print "%d is divisible by 4" % counter ... if counter % 5 == 0: ... print "%d is divisible by 5" % counter ... counter = counter + 2 ... 0 is divisible by 2 0 is divisible by 3 0 is divisible by 4 0 is divisible by 5 2 is divisible by 2 4 is divisible by 2 4 is divisible by 4 6 is divisible by 2 6 is divisible by 3 8 is divisible by 2 8 is divisible by 4 10 is divisible by 2 10 is divisible by 5 12 is divisible by 2 12 is divisible by 3 12 is divisible by 4 14 is divisible by 2 16 is divisible by 2 16 is divisible by 4 18 is divisible by 2 18 is divisible by 3 20 is divisible by 2 20 is divisible by 4 20 is divisible by 5 >>>
In the next post we will see how to handle Exceptions .
Leave a Reply