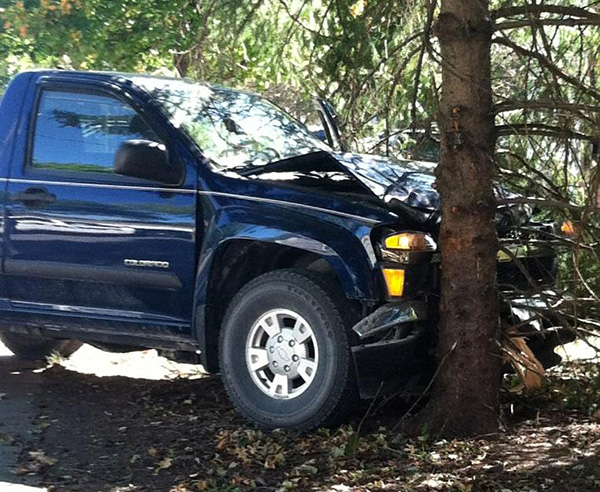
As a developer you often need to know the details of an app crash, specially if it happens on a customer’s device or any remote device. If your app is being downloaded via Play Store then you can use the existing Google services to track your crash reports. But if your app is still being tested or its a private app meant for restricted usage, then you cannot depend on Play Store to handle it for you.
There are quite a few commercial as well as free services like ACRA, Crashlytics etc. But sometimes, you dont need a full fledged service or API to do crash handling. At the very basic level, all a developer needs is the stack trace of the exception that crashed the app.
You can easily roll out your own module for handling a crash and gathering the details. This code traps any unhandled exception within your app, captures the detailed stack trace and allows a user to send the details as an email to a designated email id.
The core logic is simple – we subclass the main Application class and trap any unhandled exception, get the stack trace and call a Mailing Intent. That is all. We dont need to change any existing code anywhere else.
Create a Class file and extend it from Application.
package com.myapp.test; import android.app.Application; import android.content.Context; import android.content.Intent; import android.util.Log; /** * Created by amit on 22/4/16. */ public class TestApplication extends Application { @Override public void onCreate() { super.onCreate(); Thread.setDefaultUncaughtExceptionHandler(new Thread.UncaughtExceptionHandler() { @Override public void uncaughtException(Thread thread, Throwable ex) { handleUncaughtException(thread, ex); } }); } public void handleUncaughtException (Thread thread, Throwable e) { String stackTrace = Log.getStackTraceString(e); String message = e.getMessage(); Intent intent = new Intent (Intent.ACTION_SEND); intent.setType("message/rfc822"); intent.putExtra (Intent.EXTRA_EMAIL, new String[] {"myemail@email.com"}); intent.putExtra (Intent.EXTRA_SUBJECT, "MyApp Crash log file"); intent.putExtra (Intent.EXTRA_TEXT, stackTrace); intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK); // required when starting from Application startActivity(intent); } }
In your AndroidManifest.xml specify the name for your application tag to be the name of your Class file.
<application android:name=".TestApplication"
Whenever your application crashes, it will get caught in your custom Application class, and then it will call the defaultĀ mailing application in the device by setting up a Mail Intent. Everything in the mail will already be filled – the user just has to click on Send. Or he can even copy the mail content and save it somewhere.
Hi. I have tried the above example…I have commented the one activity in Manifest file and ran the application…but am getting the same issue ie. the app is crashed ..
Do we have to use this class in other classes?
Waiting for the Response…….please help me out š
@Kiran. Sorry I dont check my blog very often.
In android manifest you have to make sure your application is using your custom application class
If your application is still crashing directly then it is not extending the custom application class. To confirm that your Application class is being used, run the app in debug mode and put a breakpoint in the onCreate method and in the handleUncaughtException method with the custom application class.
On startup, the breakpoint in oncreate should fire. On an uncaught exception anywhere in the application, the handleUncaughtException breakpoint should fire .
Thanks it worked for me. But another issue i am facing is, once i sent the crash reports through the mail and returned to the app from email intent, backpressed is not working or the whole app is not responding.
Try to restart the app