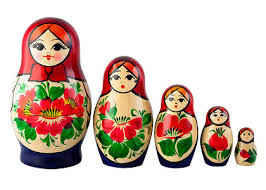
Apart from the standard sorting function which will sort the values of an array in ascending or descending order, PHP provides numerous other functions which can do sorting in different ways.
There are three points to consider for any sorting method:
- Will the same key=>value relationship be maintained after sorting?
- Will it sort on the key or the value?
- Will it let us define our own sorting logic or use an inbuilt one?
We look at 12 different sorting functions here.
sort()
Sorts the elements of an array by value in ascending order. Key=>value relationship is changed after sorting.
Original array= array(15) { [0]=> int(34) [1]=> int(67) [2]=> int(98) [3]=> int(1) [4]=> int(21) [5]=> int(90) [6]=> int(32) [7]=> int(66) [8]=> int(81) [9]=> int(46) [10]=> int(51) [11]=> int(21) [12]=> int(6) [13]=> int(99) [14]=> int(70) } Using sort() array(15) { [0]=> int(1) [1]=> int(6) [2]=> int(21) [3]=> int(21) [4]=> int(32) [5]=> int(34) [6]=> int(46) [7]=> int(51) [8]=> int(66) [9]=> int(67) [10]=> int(70) [11]=> int(81) [12]=> int(90) [13]=> int(98) [14]=> int(99) }
rsort()
Sorts the elements of an array by value in descending order. Key=>value relationship is changed after sorting.
Original array= array(15) { [0]=> int(34) [1]=> int(67) [2]=> int(98) [3]=> int(1) [4]=> int(21) [5]=> int(90) [6]=> int(32) [7]=> int(66) [8]=> int(81) [9]=> int(46) [10]=> int(51) [11]=> int(21) [12]=> int(6) [13]=> int(99) [14]=> int(70) } Using rsort() array(15) { [0]=> int(99) [1]=> int(98) [2]=> int(90) [3]=> int(81) [4]=> int(70) [5]=> int(67) [6]=> int(66) [7]=> int(51) [8]=> int(46) [9]=> int(34) [10]=> int(32) [11]=> int(21) [12]=> int(21) [13]=> int(6) [14]=> int(1) }
usort()
The sorting will occur using a user defined function comparing the values. Key=>value relationship is changed after sorting. The user-defined function has to take in two parameters, $a and $b representing the two values to compare and return an integer value as follows:
- Less than zero – means $a < $b
- Zero means $a = $b
- More than zero means $a > $b
Original array= array(15) { [0]=> int(34) [1]=> int(67) [2]=> int(98) [3]=> int(1) [4]=> int(21) [5]=> int(90) [6]=> int(32) [7]=> int(66) [8]=> int(81) [9]=> int(46) [10]=> int(51) [11]=> int(21) [12]=> int(6) [13]=> int(99) [14]=> int(70) } Using usort() array(15) { [0]=> int(1) [1]=> int(6) [2]=> int(21) [3]=> int(21) [4]=> int(32) [5]=> int(34) [6]=> int(46) [7]=> int(51) [8]=> int(66) [9]=> int(67) [10]=> int(70) [11]=> int(81) [12]=> int(90) [13]=> int(98) [14]=> int(99) } Function used: function cmp($a, $b) { if ($a == $b) { return 0; } return ($a < $b) ? -1 : 1; }
asort()
Sorts the elements of an array by value in ascending order. Key=>value relationship is maintained after sorting.
Original array= array(15) { [0]=> int(34) [1]=> int(67) [2]=> int(98) [3]=> int(1) [4]=> int(21) [5]=> int(90) [6]=> int(32) [7]=> int(66) [8]=> int(81) [9]=> int(46) [10]=> int(51) [11]=> int(21) [12]=> int(6) [13]=> int(99) [14]=> int(70) } Using asort() array(15) { [3]=> int(1) [12]=> int(6) [4]=> int(21) [11]=> int(21) [6]=> int(32) [0]=> int(34) [9]=> int(46) [10]=> int(51) [7]=> int(66) [1]=> int(67) [14]=> int(70) [8]=> int(81) [5]=> int(90) [2]=> int(98) [13]=> int(99) }
arsort()
Sorts the elements of an array by value in descending order. Key=>value relationship is maintained after sorting.
Original array= array(15) { [0]=> int(34) [1]=> int(67) [2]=> int(98) [3]=> int(1) [4]=> int(21) [5]=> int(90) [6]=> int(32) [7]=> int(66) [8]=> int(81) [9]=> int(46) [10]=> int(51) [11]=> int(21) [12]=> int(6) [13]=> int(99) [14]=> int(70) } Using arsort() array(15) { [13]=> int(99) [2]=> int(98) [5]=> int(90) [8]=> int(81) [14]=> int(70) [1]=> int(67) [7]=> int(66) [10]=> int(51) [9]=> int(46) [0]=> int(34) [6]=> int(32) [4]=> int(21) [11]=> int(21) [12]=> int(6) [3]=> int(1) }
ksort()
Sort array by the keys in ascending order instead of values. Key=>value relationship is maintained after sorting.
Original array= array(5) { ["c"]=> int(10) ["a"]=> int(9) ["b"]=> int(56) ["e"]=> int(24) ["d"]=> int(41) } Using ksort() array(5) { ["a"]=> int(9) ["b"]=> int(56) ["c"]=> int(10) ["d"]=> int(41) ["e"]=> int(24) }
natsort()
Sort array by values in ascending order using natural ordering instead of strict ASCII ordering. Key=>value relationship is maintained after sorting.
Original array= array(6) { [0]=> string(5) "test1" [1]=> string(6) "test15" [2]=> string(5) "Test2" [3]=> string(5) "test3" [4]=> string(6) "Test13" [5]=> string(5) "test5" } Using natsort() array(6) { [2]=> string(5) "Test2" [4]=> string(6) "Test13" [0]=> string(5) "test1" [3]=> string(5) "test3" [5]=> string(5) "test5" [1]=> string(6) "test15" }
natcasesort()
Sort array by values in ascending order using natural ordering in a case-insensitive way instead of strict ASCII ordering. Key=>value relationship is maintained after sorting.
Original array= array(6) { [0]=> string(5) "test1" [1]=> string(6) "test15" [2]=> string(5) "Test2" [3]=> string(5) "test3" [4]=> string(6) "Test13" [5]=> string(5) "test5" } Using natcasesort() array(6) { [0]=> string(5) "test1" [2]=> string(5) "Test2" [3]=> string(5) "test3" [5]=> string(5) "test5" [4]=> string(6) "Test13" [1]=> string(6) "test15" }
array_multisort()
Sort multi-dimensional arrays in ascending or descending order by values. Key=>value relationship is not maintained after sorting.
Original array= array(2) { [0]=> array(5) { [0]=> int(20) [1]=> int(11) [2]=> int(101) [3]=> int(100) [4]=> int(98) } [1]=> array(5) { [0]=> int(1) [1]=> int(12) [2]=> int(0) [3]=> int(3) [4]=> int(9) } } Calling sort as array_multisort($multi[0], SORT_ASC, $multi[1], SORT_DESC); Using array_multisort() array(2) { [0]=> array(5) { [0]=> int(11) [1]=> int(20) [2]=> int(98) [3]=> int(100) [4]=> int(101) } [1]=> array(5) { [0]=> int(12) [1]=> int(1) [2]=> int(9) [3]=> int(3) [4]=> int(0) } }
uksort()
Sort array by the keys using a user-defined function. Key=>value relationship is maintained after sorting.
The user-defined function has to take in two parameters, $a and $b representing the two values to compare and return an integer value as follows:
- Less than zero – means $a < $b
- Zero means $a = $b
- More than zero means $a > $b
Original array(6) { ["ax"]=> int(100) ["bxb"]=> int(500) ["ya"]=> int(100) ["xp"]=> int(200) ["yy"]=> int(100) ["xm"]=> int(40) } Using uksort() array(6) { ["xp"]=> int(200) ["xm"]=> int(40) ["ax"]=> int(100) ["bxb"]=> int(500) ["yy"]=> int(100) ["ya"]=> int(100) } Function used: { if (strpos($a, "x") !== FALSE) { return 0; } else return 1; }
Leave a Reply