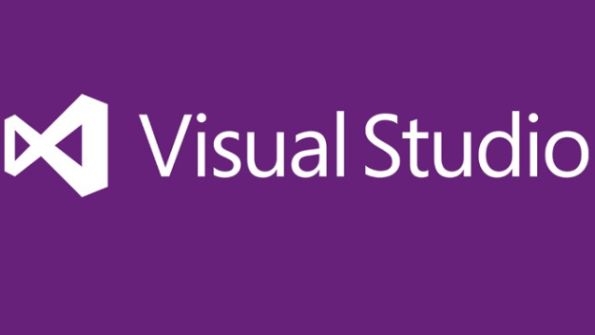
For those who use Visual Studio (VS) on a regular basis, they know that VS has hundreds of options and features but most people only use a small subset of them on a regular basis. It is always wise to know and explore all the capabilities of your IDE, because they could save you a lot of time and trouble and make you more productive.
Here we are going to look at some interesting options which are available under the Edit Menu, specifically:
- Cycle Clipboard Ring
- Paste Special
- Insert File As Text
Cycle Clipboard Ring
Normally when you do Ctrl-C and Ctrl-V multiple times, whatever data you copied last is what is pasted. So for instance, if you have three lines of code:
int x = 0 int y = 0 int z = 0
If you copy each line separately starting from x to y, then when you press Ctrl-V you will get int z=0
But if you press Ctrl-Shift-V successively then it will cycle through the three lines in the clipboard and you can choose which one to paste.
So pressing Ctrl-Shift-V once, we get int z = 0.
Pressing it again, we get int y = 0
Pressing it again we get int x = 0
Paste Special
Paste Special has two suboptions:
- Paste JSON as Classes
- Paste XML as Classes
This is a very useful tool to have, as it automatically converts a JSON string or an XML string copied into the clipboard into relevant C# classes with the correct methods and attributes.
Here is an example of JSON conversion:
[ { color: "red", value: "#f00" }, { color: "green", value: "#0f0" }, { color: "blue", value: "#00f" }, { color: "cyan", value: "#0ff" }, { color: "magenta", value: "#f0f" }, { color: "yellow", value: "#ff0" }, { color: "black", value: "#000" } ]
When you do a Paste Special with the above data, it becomes:
public class Rootobject { public Class1[] Property1 { get; set; } } public class Class1 { public string color { get; set; } public string value { get; set; } }
Here is an example of XML conversion:
When you do a Paste Special with the above data, it becomes:
/// [System.Xml.Serialization.XmlTypeAttribute(AnonymousType = true)] [System.Xml.Serialization.XmlRootAttribute(Namespace = "", IsNullable = false)] public partial class catalog { private catalogProduct productField; /// public catalogProduct product { get { return this.productField; } set { this.productField = value; } } } /// [System.Xml.Serialization.XmlTypeAttribute(AnonymousType = true)] public partial class catalogProduct { private catalogProductCatalog_item[] catalog_itemField; private string descriptionField; private string product_imageField; /// [System.Xml.Serialization.XmlElementAttribute("catalog_item")] public catalogProductCatalog_item[] catalog_item { get { return this.catalog_itemField; } set { this.catalog_itemField = value; } } /// [System.Xml.Serialization.XmlAttributeAttribute()] public string description { get { return this.descriptionField; } set { this.descriptionField = value; } } /// [System.Xml.Serialization.XmlAttributeAttribute()] public string product_image { get { return this.product_imageField; } set { this.product_imageField = value; } } } /// [System.Xml.Serialization.XmlTypeAttribute(AnonymousType = true)] public partial class catalogProductCatalog_item { private string item_numberField; private decimal priceField; private catalogProductCatalog_itemSize[] sizeField; private string genderField; /// public string item_number { get { return this.item_numberField; } set { this.item_numberField = value; } } /// public decimal price { get { return this.priceField; } set { this.priceField = value; } } /// [System.Xml.Serialization.XmlElementAttribute("size")] public catalogProductCatalog_itemSize[] size { get { return this.sizeField; } set { this.sizeField = value; } } /// [System.Xml.Serialization.XmlAttributeAttribute()] public string gender { get { return this.genderField; } set { this.genderField = value; } } } /// [System.Xml.Serialization.XmlTypeAttribute(AnonymousType = true)] public partial class catalogProductCatalog_itemSize { private catalogProductCatalog_itemSizeColor_swatch[] color_swatchField; private string descriptionField; /// [System.Xml.Serialization.XmlElementAttribute("color_swatch")] public catalogProductCatalog_itemSizeColor_swatch[] color_swatch { get { return this.color_swatchField; } set { this.color_swatchField = value; } } /// [System.Xml.Serialization.XmlAttributeAttribute()] public string description { get { return this.descriptionField; } set { this.descriptionField = value; } } } /// [System.Xml.Serialization.XmlTypeAttribute(AnonymousType = true)] public partial class catalogProductCatalog_itemSizeColor_swatch { private string imageField; private string valueField; /// [System.Xml.Serialization.XmlAttributeAttribute()] public string image { get { return this.imageField; } set { this.imageField = value; } } /// [System.Xml.Serialization.XmlTextAttribute()] public string Value { get { return this.valueField; } set { this.valueField = value; } } }
Insert File As Text
This option is self-explanatory. Instead of having to open a file with text data externally and manually copying and pasting the contents into your IDE, you can choose Insert File as Text, navigate to the file and click on it. The contents of the file will be automatically copied into your current cursor position.
Leave a Reply