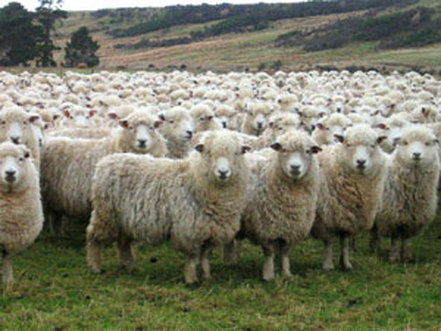
The code below is a single function which can be plugged into any page to capture and store all the HTTP headers, available in the page request, as an associative array. The function also dumps the data to the page . No external library is required – this is pure javascript and thus runs in all browsers.
A lot of people try to obtain the client IP address in javascript, but that is not possible. You need either an external service like MaxMind or a serverside script to get the client IP address.
To display the data the function code assumes there is a DIV defined in the page with an id of “dump”.
function getHeaders() { var req = new XMLHttpRequest(); req.open('GET', document.location, false); req.send(null); // associate array to store all values var data = new Object(); // get all headers in one call and parse each item var headers = req.getAllResponseHeaders().toLowerCase(); var aHeaders = headers.split('\n'); var i =0; for (i= 0; i < aHeaders.length; i++) { var thisItem = aHeaders[i]; var key = thisItem.substring(0, thisItem.indexOf(':')); var value = thisItem.substring(thisItem.indexOf(':')+1); data[key] = value; } // get referer var referer = document.referrer; data["Referer"] = referer; //get useragent var useragent = navigator.userAgent; data["UserAgent"] = useragent; //extra code to display the values in html var display = ""; for(var key in data) { if (key != "") display += "<b>" + key + "</b> : " + data[key] + "<br>"; } document.getElementById("dump").innerHTML = display; }
You can see the code in action here.
Thanks
It helped me a lot
Hi, Can you try this scenario which i have posted in below URL and let me know whether we can able to get the referrrer URL?
https://social.msdn.microsoft.com/Forums/sharepoint/en-US/1feecdd9-10f4-4674-8e80-943d01990f9f/how-to-get-my-referrer-url-using-jquery-in-sharepoint-2013-site?forum=sharepointgeneral