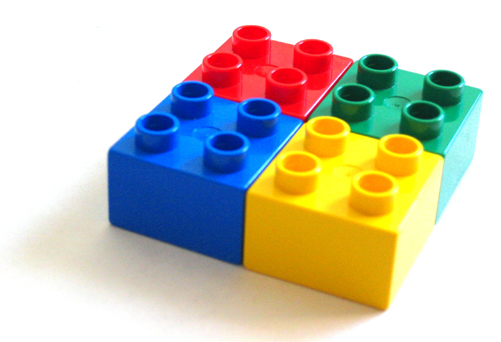
We are now ready to create our first extension and as expected we will call our extension “helloworld”.
PHP-CPP provides us with a template project to help create our own extension. You will find this in the PHP-CPP/Examples folder . Among the several example projects, there is one called EmptyExtension. This project contains three files:
- yourextension.ini – the file which tells PHP that your extension is available for loading
- Makefile – Standard makefile for g++
- main.cpp -The main c++ file where you will add your extension code
STEP 1 – Make your own folder with the example files
- Copy the folder EmptyExtension and put it where your want to do your development work. Rename the folder to helloworld
- Rename yourextension.ini to helloworld.ini
STEP 2 – Changes to Makefile
- Run php-config from the command prompt. Look for the line –extension-dir [<path to extension dir>]
- Locate conf.d for your php installation. Most likely this will be in /etc/php5/cli/conf.d
- Open Makefile in your editor.
- Change the line NAME = yourextension to NAME = helloworld
- Change the line INI_DIR = /etc/php5/mods-available/ to the path of conf.d eg. INI_DIR = /etc/php5/cli/conf.d
STEP 3- Changes in .ini
- Open helloworld.ini in your editor
- Change the line extension=yourextension.so to extension=helloworld.so
STEP 4- Changes to main.cpp
For now we will just change the name of the extension in the sample code.
- Change the line static Php::Extension extension(“yourextension”, “1.0”); to static Php::Extension extension(“helloworld”, “1.0”);
So your main.cpp should be looking as below:
#include <phpcpp.h> /** * tell the compiler that the get_module is a pure C function */ extern "C" { /** * Function that is called by PHP right after the PHP process * has started, and that returns an address of an internal PHP * strucure with all the details and features of your extension * * @return void* a pointer to an address that is understood by PHP */ PHPCPP_EXPORT void *get_module() { // static(!) Php::Extension object that should stay in memory // for the entire duration of the process (that's why it's static) static Php::Extension extension("helloworld", "1.0"); // @todo add your own functions, classes, namespaces to the extension // return the extension return extension; } }
STEP-5 COMPILE AND MAKE
From the command prompt, run make
and then sudo make install
At this stage, helloworld.so should have been copied into your php runtime directory and in conf.d
The runtime folder will be the same as the extension dir as shown in the output of php-config. which you did earlier.
To check if the extension helloworld.so is now part of the php extension library, type php -i | grep helloworld
This completes the process of creating a custom PHP extension. We have made an extension, though it is not doing anything yet. In the next Part we will start adding code to the extension and see it run in your PHP code.
Please bear in mind that currently this extension is only runnable in the CLI version of PHP and is not part of the web-server based PHP library like Apache. So only PHP scripts run from the command line will be able to use the extension. We will look into using the extension in a web environment later.
Leave a Reply