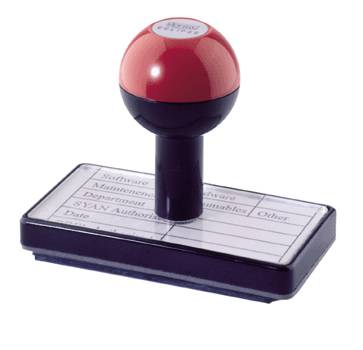
Function to add a text watermark in C#. It is a generic function which can be used in either a desktop application or an ASP.NET website. It works with JPG and PNG files.
GIF files are also processed without any errors or Exceptions but no watermark is added. This is due to the common issue “A Graphics object cannot be created from an image that has an indexed pixel format.” There are ways around this problem by first converting the image object into a generic bitmap, doing the transformations and then saving it asĀ GIF, but somehow that approach didnt work either. If anyone has a solution to it, I will be glad to modify the code to incorporate it.
The test images are shown below:
Example JPG Image
Example PNG image
The code is given below:
using System.Drawing; using System.Drawing.Imaging; using System.IO; /// <summary> /// Add a text watermark to an image /// </summary> /// <param name="sourceImage">path to source image</param> /// <param name="text">text to add as a watermark</param> /// <param name="targetImage">path to the modified image</param> /// <param name="fmt">ImageFormat type</param> public void watermarkImage(string sourceImage, string text, string targetImage, ImageFormat fmt) { try { // open source image as stream and create a memorystream for output FileStream source = new FileStream(sourceImage, FileMode.Open); Stream output = new MemoryStream(); Image img = Image.FromStream(source); // choose font for text Font font = new Font("Arial", 20, FontStyle.Bold, GraphicsUnit.Pixel); //choose color and transparency Color color = Color.FromArgb(100, 255, 0, 0); //location of the watermark text in the parent image Point pt = new Point(10, 5); SolidBrush brush = new SolidBrush(color); //draw text on image Graphics graphics = Graphics.FromImage(img); graphics.DrawString(text, font, brush, pt); graphics.Dispose(); //update image memorystream img.Save(output, fmt); Image imgFinal = Image.FromStream(output); //write modified image to file Bitmap bmp = new System.Drawing.Bitmap(img.Width, img.Height, img.PixelFormat); Graphics graphics2 = Graphics.FromImage(bmp); graphics2.DrawImage(imgFinal, new Point(0, 0)); bmp.Save(targetImage, fmt); imgFinal.Dispose(); img.Dispose(); } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void Form1_Load(object sender, EventArgs e) { string sourceImage = "d:\\junk\\Watermark\\clouds.jpg"; string targetImage = "d:\\junk\\watermark\\watermarked.jpg"; watermarkImage(sourceImage, "This is a watermark", targetImage, ImageFormat.Jpeg); sourceImage = "d:\\junk\\Watermark\\house.png"; targetImage = "d:\\junk\\watermark\\watermarked.png"; watermarkImage(sourceImage, "This is a watermark", targetImage, ImageFormat.Png); sourceImage = "d:\\junk\\Watermark\\garden.gif"; targetImage = "d:\\junk\\watermark\\watermarked.gif"; watermarkImage(sourceImage, "This is a watermark", targetImage, ImageFormat.Gif); }
Leave a Reply