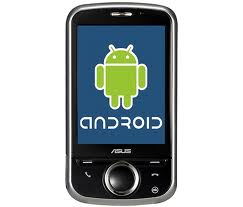
A small utility to display the list of Sensors present in an Android phone.
For those who are not interested in the code, the installable apk file is provided as a downloadable link at the end of this article.
The Activity class
package com.example.listsensors; import java.util.List; import android.os.Bundle; import android.hardware.Sensor; import android.hardware.SensorManager; import android.app.Activity; import android.content.Context; import android.view.Menu; import android.widget.TextView; public class MainActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); SensorManager mgr = (SensorManager) getSystemService(Context.SENSOR_SERVICE); List sensors = mgr.getSensorList(Sensor.TYPE_ALL); String data = ""; for(int i =0; i < sensors.size(); i++) { Sensor s = (Sensor) sensors.get(i); data += s.getName() + "\r\n"; } TextView view = (TextView) findViewById(R.id.txtList); view.setText(data); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main, menu); return true; } }
The Layout xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".MainActivity" > <TextView android:id="@+id/txtList" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/hello_world" /> </RelativeLayout>
The installable APK file can be downloaded from here.
Be sure to allow Unknown Sources in your Settings->Applications, since this app is not part of the Google Play Store.
Here is a screenshot of how it looks. (Sorry not very clear).
Leave a Reply