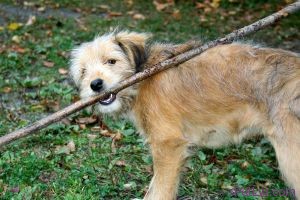
One good built-in check in Android development is the fact if any network operation is done, the compiler flags an error if it is being executed in the main thread. So any code which is trying to access something outside the device, needs to be in a separate thread.
AsyncTask is an excellent choice for this, because it is meant primarily to execute some time consuming background task and then update the UI thread in a simple manner.
Below we see how to fetch data from a URL and then update the UI once it has completed the network operation.
CALLING ASYNCTASK AS INNER CLASS
First the AsyncTask object has to be defined. Here we define it as an inner class. This is the preferred method, though it does create messy code if you have a lot of code to write in the inner class. From a design point of view, it is better to implement AsyncTask as an external class and then use a Listener in the calling class to interact with it. However this requires that the entire UI object structure needs to be passed between the two classes.
The whole point of using ASyncTask is that it is meant to work with the UI. So calling it as an inner class allows it access to the UI structure of the outer class without having to pass arguments.
The code is given below:
public class SomeActivity extends Activity { AsyncTask<Void, Void, Void> mTask; // define this at the class level public void test() { mTask = new AsyncTask<Void, Void, Void> () { @Override protected Void doInBackground(Void... params) { try { jsonString = getFromFile(); if (jsonString == null || jsonString == "") { jsonString = getJsonFromServer("some url here"); } } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } return null; } @Override protected void onPostExecute(Void result) { super.onPostExecute(result); try { if (jsonString != null && jsonString != "") // do something here } catch (IOException ie){ //ie.printStackTrace(); } } }; // mtask // call the asynctask defined above mTask.execute(); } public static String getJsonFromServer(String url) throws IOException { BufferedReader inputStream = null; URL jsonUrl = new URL(url); URLConnection dc = jsonUrl.openConnection(); dc.setConnectTimeout(5000); dc.setReadTimeout(5000); inputStream = new BufferedReader(new InputStreamReader( dc.getInputStream())); // read the JSON results into a string String jsonResult = inputStream.readLine(); inputStream.close(); return jsonResult; } }
It is using a generic function getJsonFromServer() to do the actual fetching of data. Please note that the function is just getting the data as a string – its not really doing any json processing as the name suggests.
MORE ON ASYNCTASK
AsyncTask has four main methods which can be overridden:
- onPreExecute() – this can access the UI thread before the processing starts. Generally used to show a progress bar or something similar before starting the task
- doInBackground() – this is the main workhorse function and does the actual background task. This method is not supposed to try and access the UI
- onProgressUpdate() – invoked on the UI thread after a call to publishProgress(). This is generally used to keep the UI updated as to whats happening in the task
- onPostExecute() – this is called after doBackground() finishes. This method can access the UI and update it as required
The doInBackground() method takes in 3 arguments, all of which are optional.
- Params: defines the Type of the parameters which are passed when the Task is created
- Progress: the Type of the progress units published during the background computation.
- Result: the Type of the result after the completion of this method
If no data needs to be passed then it is called as AsyncTask<Void, Void, Void> . This is how the above sample code is working. It needs no arguments to be passed.
If a String needs to be passed to the task and the returning result is also a String then it is called as AsyncTask<String, Void, String>
The first argument , if passed is usually an array of that data type meant for the doInBackground() method
protected String doInBackground(String... params) { String url=params[0]; String retVal = ""; /// do processing here return retVal; } protected void onPostExecute(String result) { super.onPostExecute(result); // do something here }
Leave a Reply