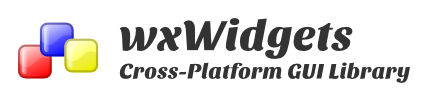
OVERVIEW
We use wxBitmapButton when we want to show an image on a button. We can also show text along with the image, in which case we can set the text alignment. The code below uses 3 images which are toggled on the same button every time it is clicked.
SAMPLE CODE
bitmapbutton.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class BitmapButton: public wxFrame {
public:
BitmapButton(const wxString &title);
void onClick(wxCommandEvent &event);
private:
wxBitmapButton *mBtn;
int mCurrImage;
};
bitmapbutton.cpp
#include "bitmapbutton.h"
BitmapButton::BitmapButton(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,200)) {
wxPanel *panel = new wxPanel(this, -1);
wxBoxSizer *vbox = new wxBoxSizer(wxVERTICAL);
panel->SetSizer(vbox);
mBtn = new wxBitmapButton(panel, -1, wxBitmap(wxT("1.png"), wxBITMAP_TYPE_PNG), wxDefaultPosition);
vbox->Add(mBtn, 1, wxEXPAND);
mCurrImage = 0;
mBtn->Bind(wxEVT_BUTTON, &BitmapButton::onClick, this);
Center();
}
void BitmapButton::onClick(wxCommandEvent &event) {
mCurrImage ++;
if (mCurrImage > 2)
mCurrImage = 0;
if (mCurrImage == 0)
mBtn->SetBitmapLabel(wxBitmap(wxT("1.png"), wxBITMAP_TYPE_PNG));
else if (mCurrImage == 1)
mBtn->SetBitmapLabel(wxBitmap(wxT("2.png"), wxBITMAP_TYPE_PNG));
else if (mCurrImage == 2)
mBtn->SetBitmapLabel(wxBitmap(wxT("3.png"), wxBITMAP_TYPE_PNG));
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "bitmapbutton.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
BitmapButton *app = new BitmapButton(wxT("BitmapButton"));
app->Show(true);
}
The output is shown below
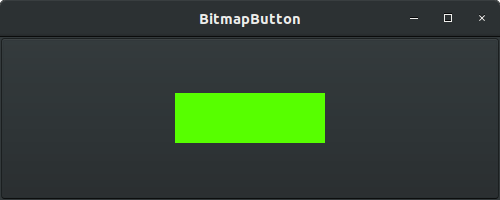
The images used can be downloaded from below
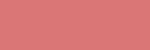
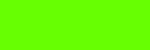
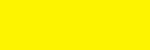
Leave a Reply