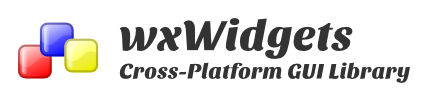
OVERVIEW
We now create a custom dialog which takes the code from the two previous sections and puts them together to show a dialog where you can load a text file in an editor and then change the font of the editor.
SAMPLE CODE
dialog-custom.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class DialogCustom: public wxFrame {
public:
DialogCustom(const wxString& title);
void OnFileClick(wxCommandEvent &event);
void OnFontClick(wxCommandEvent &event);
private:
wxTextCtrl *mTxt;
};
dialog-custom.cpp
#include <wx/fontdlg.h>
#include "dialog-custom.h"
DialogCustom::DialogCustom(const wxString& title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,400)) {
wxPanel *panelMain = new wxPanel(this, -1);
wxBoxSizer *vbox = new wxBoxSizer(wxVERTICAL);
panelMain->SetSizer(vbox);
wxPanel *panelButtons = new wxPanel(panelMain,-1);
wxBoxSizer *hbox = new wxBoxSizer(wxHORIZONTAL);
panelButtons->SetSizer(hbox);
wxButton *btn1 = new wxButton(panelButtons, -1, wxT("Choose Text File"));
wxButton *btn2 = new wxButton(panelButtons, -1, wxT("Choose Font"));
hbox->Add(btn1, 1, wxALL | wxALIGN_LEFT, 5);
hbox->Add(btn2, 1, wxALL , 5);
mTxt = new wxTextCtrl(panelMain, -1, wxT(""), wxPoint(-1,-1), wxSize(-1,-1), wxTE_MULTILINE);
vbox->Add(panelButtons, 0, wxALL ,5);
vbox->Add(mTxt, 1, wxALL | wxEXPAND | wxCENTER, 5);
btn1->Bind(wxEVT_BUTTON, &DialogCustom::OnFileClick, this);
btn2->Bind(wxEVT_BUTTON, &DialogCustom::OnFontClick, this);
Center();
}
void DialogCustom::OnFileClick(wxCommandEvent &event) {
wxFileDialog *dlg = new wxFileDialog(this, wxT("Open Text File"), wxT(""), wxT(""), wxT("txt files (*.txt)|*.txt"),
wxFD_OPEN | wxFD_FILE_MUST_EXIST);
int retVal = dlg->ShowModal();
if (retVal == wxID_OK) {
wxString path = dlg->GetPath();
mTxt->LoadFile(path);
}
}
void DialogCustom::OnFontClick(wxCommandEvent &event) {
wxFontDialog *dlg = new wxFontDialog(this);
int retVal = dlg->ShowModal();
if (retVal == wxID_OK) {
mTxt->SetFont(dlg->GetFontData().GetChosenFont());
}
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "dialog-custom.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
DialogCustom *app = new DialogCustom(wxT("Custom Dialog "));
app->Show(true);
}
The output is shown below
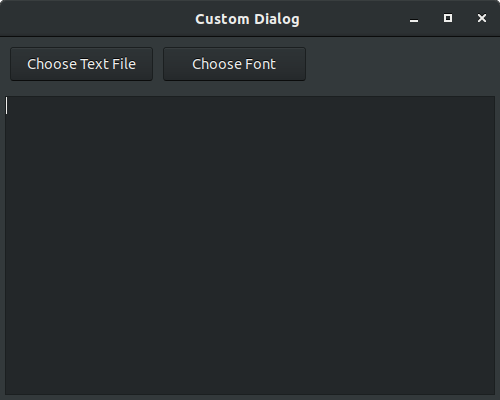
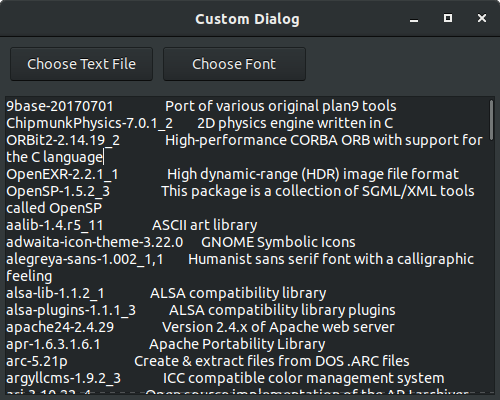
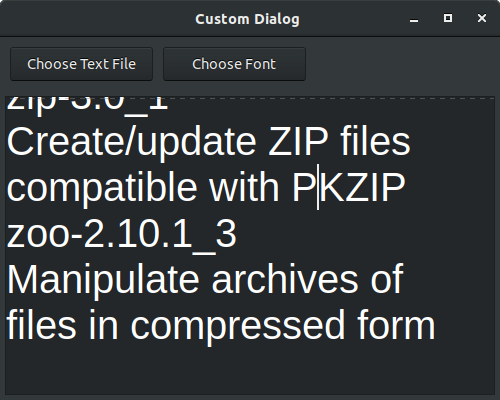
Leave a Reply