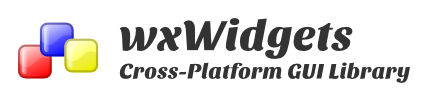
OVERVIEW
We take the example from the previous section and implement the same using Bind.
SAMPLE CODE
events-bind.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class EventsBind: public wxFrame {
public:
EventsBind(const wxString& title);
void OnClick(wxCommandEvent &event);
void OnText(wxCommandEvent &event);
private:
wxStaticText *m_lbl;
wxTextCtrl *m_edit;
};
const int ID_BTN_1 = 101;
const int ID_BTN_2 = 102;
const int ID_BTN_3 = 103;
const int ID_TXT_1 = 104;
events-bind.cpp
#include "events-bind.h"
EventsBind::EventsBind(const wxString& title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(500,200)) {
wxPanel *panelMain = new wxPanel(this, -1);
wxBoxSizer *vbox = new wxBoxSizer(wxVERTICAL);
panelMain->SetSizer(vbox);
wxPanel *panelBase = new wxPanel(panelMain, -1);
wxBoxSizer *hbox = new wxBoxSizer(wxHORIZONTAL);
panelBase->SetSizer(hbox);
wxButton *btn1 = new wxButton(panelBase, ID_BTN_1, wxT("Button 1"));
wxButton *btn2 = new wxButton(panelBase, ID_BTN_2, wxT("Button 2"));
wxButton *btn3 = new wxButton(panelBase, ID_BTN_3, wxT("Button 3"));
m_edit = new wxTextCtrl(panelBase, ID_TXT_1, wxT(""));
hbox->Add(btn1, 1, wxALL,5);
hbox->Add(btn2, 1, wxALL,5);
hbox->Add(btn3, 1, wxALL,5);
hbox->Add(m_edit, 2, wxALL,5);
vbox->Add(panelBase,1, wxALL,2);
m_lbl = new wxStaticText(panelMain, -1, wxT("show event handling here"));
vbox->Add(m_lbl, 1, wxALL | wxALIGN_CENTER);
btn1->Bind(wxEVT_BUTTON, &EventsBind::OnClick, this);
btn2->Bind(wxEVT_BUTTON, &EventsBind::OnClick, this);
btn3->Bind(wxEVT_BUTTON, &EventsBind::OnClick, this);
m_edit->Bind(wxEVT_TEXT, &EventsBind::OnText, this);
Center();
}
void EventsBind::OnClick(wxCommandEvent &event) {
wxString strId = wxString::Format(wxT("%d"), event.GetId());
strId.Append(wxString(" clicked"));
m_lbl->SetLabel(strId);
}
void EventsBind::OnText(wxCommandEvent &event) {
m_lbl->SetLabel(m_edit->GetValue());
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif
class Main: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
#include "events-bind.h"
IMPLEMENT_APP(Main)
bool Main::OnInit() {
EventsBind *app = new EventsBind(wxT("Events Bind"));
app->Show(true);
}
The output is shown below
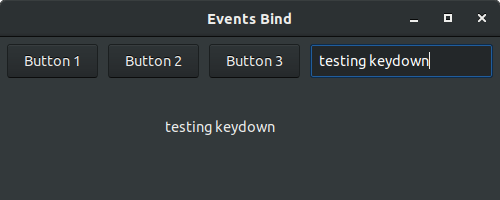
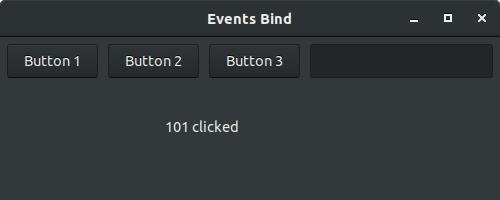
Leave a Reply