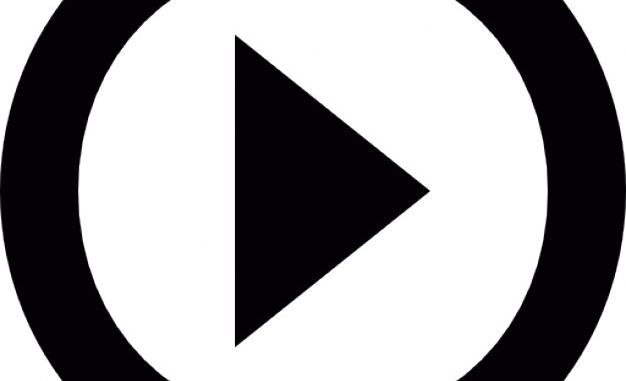
This is article 15 of the YouTube API With PHP series.
A Channel Banner is the image which shows up the Channel homepage in the page header. You can only set one image for a banner. This API resource has only one function available.
1.insert
This API function will upload an image and assign it as the Channel banner image. The following restrictions apply:
- The image has to be a valid jpg pr png.
- It should have a minimum size of 2048×1152 pixels. Ideally it should be 2560px by 1440px .
- It should have an aspect ratio of 16:9
- It cannot exceed 6 Mb in filesize.
Updating an image as the Channel Banner requires two API calls:
- The first API call channelBanner.insert will upload the image. A successful upload will return the URL of the uploaded image.
- This URL will then have to be updated in the Channel by calling the channel.update API call.
This API requires user authentication so you can only update the banner of your own channel. The access token is passed in the request header and not as part of the request URL.
The Request URLs are
POST https://www.googleapis.com/upload/youtube/v3/channelBanners/insert PUT https://www.googleapis.com/youtube/v3/channels
Parameters:
- key (string) required. Your API key
- onBehalfOfContentOwner (string) optional. This is relevant only for YouTube Channel Partners. For this parameter, the API request URL should have user authentication.We will not be exploring this option.
Example Requests
Update the channel banner of the current authenticated user
https://www.googleapis.com/upload/youtube/v3/channelBanners/insert?key=xxxx https://www.googleapis.com/youtube/v3/channels?part=brandingSettings&key=xxx&access_token=xxx
Response
A successful request will return a json encoded ChannelBanner resource which is shown below.
{ "kind": "youtube#channelBannerResource", "etag": etag, "url": string }
The Response components are explained below:
- kind (string) “youtube#channelBannerResource.”
- etag (string) See ETags Explained section
- url (string) url of the banner image
Sample code to update the channel banner:
<?php error_reporting(E_ALL ^ E_NOTICE ^ E_WARNING ^ E_DEPRECATED); set_time_limit(60 * 3); session_start(); $clientId = "***"; $clientSecret = "**"; $g_youtubeDataAPIKey = "**"; $channelId = "UCnXmfpAZ1rLsg0Goh0bBHUA"; $_SESSION["code_id"] = $_SERVER["PHP_SELF"]; if ($_SESSION["access_token"] == null || $_SESSION["access_token"] == "") { // check for oauth response header("Location: ../../init-login.php"); exit; } $accessToken = $_SESSION["access_token"]; // load image file contents $fh = fopen("channelbanner.png", "r"); if (!$fh) exit("Could not open image file "); fclose($fh); $fileData = file_get_contents("channelbanner.png"); // prepare curl for actual image file upload $url = "https://www.googleapis.com/upload/youtube/v3/channelBanners/insert?key=" . $g_youtubeDataAPIKey; $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_HTTPHEADER=>array('Content-Type: application/octet-stream', 'Authorization: OAuth ' . $accessToken, 'Content-Length: ' . filesize("channelbanner.png") ), CURLOPT_RETURNTRANSFER => 1, CURLOPT_URL => $url, CURLOPT_USERAGENT => 'YouTube API Tester', CURLOPT_SSL_VERIFYPEER => 1, CURLOPT_SSL_VERIFYHOST=> 0, CURLOPT_CAINFO => "../../cert/cacert.pem", CURLOPT_CAPATH => "../../cert/cacert.pem", CURLOPT_FOLLOWLOCATION => TRUE, CURLOPT_CUSTOMREQUEST=>"POST", CURLOPT_POSTFIELDS=>$fileData )); $resp = curl_exec($curl); curl_close($curl); if ($resp) { $json = json_decode($resp); $imageUrl = $json->url; } if ($imageUrl == null) exit("Error could not get image url"); else { echo("Image url:" . $imageUrl . "<br><br>"); // run the channel Update API call to update this url in the channel $image = new Image(); $image->bannerExternalUrl = $imageUrl; $brandingSettings = new BrandingSettings(); $brandingSettings->image = $image; $channel = new ChannelResource(); $channel->id = $channelId; $channel->brandingSettings = $brandingSettings; $data = json_encode($channel); $url = "https://www.googleapis.com/youtube/v3/channels?part=brandingSettings&key=" . $g_youtubeDataAPIKey . "&access_token=" . $accessToken; $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_HTTPHEADER=>array('Content-Type: application/json' ), CURLOPT_RETURNTRANSFER => 1, CURLOPT_URL => $url, CURLOPT_USERAGENT => 'YouTube API Tester', CURLOPT_SSL_VERIFYPEER => 1, CURLOPT_SSL_VERIFYHOST=> 0, CURLOPT_CAINFO => "../../cert/cacert.pem", CURLOPT_CAPATH => "../../cert/cacert.pem", CURLOPT_FOLLOWLOCATION => TRUE, CURLOPT_CUSTOMREQUEST=>"PUT", CURLOPT_POSTFIELDS=>$data )); $resp = curl_exec($curl); curl_close($curl); var_dump($resp); } //////////////////// class ChannelResource { public $id; } class BrandingSettings { public $image; } class Image { public $bannerExternalUrl; } ?>
Here is the output:
Image url:https://lh3.googleusercontent.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/s0-d/channels4_banner
string(3498) “{ “kind”: “youtube#channel”, “etag”: “\”m2yskBQFythfE4irbTIeOgYYfBU/I5p8iEauGbe17rA6tU4X_0vs0W4\””, “id”: “UCnXmfpAZ1rLsg0Goh0bBHUA”, “brandingSettings”: { “channel”: { “title”: “Amit Sengupta”, “defaultTab”: “Featured”, “profileColor”: “#000000”, “defaultLanguage”: “en” }, “image”: { “bannerImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w1060-fcrop64=1,00005a57ffffa5a8-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerMobileImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w640-fcrop64=1,32b75a57cd48a5a8-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerTabletLowImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w1138-fcrop64=1,00005a57ffffa5a8-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerTabletImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w1707-fcrop64=1,00005a57ffffa5a8-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerTabletHdImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w2276-fcrop64=1,00005a57ffffa5a8-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerTabletExtraHdImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w2560-fcrop64=1,00005a57ffffa5a8-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerMobileLowImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w320-fcrop64=1,32b75a57cd48a5a8-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerMobileMediumHdImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w960-fcrop64=1,32b75a57cd48a5a8-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerMobileHdImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w1280-fcrop64=1,32b75a57cd48a5a8-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerMobileExtraHdImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w1440-fcrop64=1,32b75a57cd48a5a8-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerTvImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w2120-fcrop64=1,00000000ffffffff-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerTvLowImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w854-fcrop64=1,00000000ffffffff-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerTvMediumImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w1280-fcrop64=1,00000000ffffffff-nd-c0xffffffff-rj-k-no/channels4_banner”, “bannerTvHighImageUrl”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w1920-fcrop64=1,00000000ffffffff-nd-c0xffffffff-rj-k-no/channels4_banner” }, “hints”: [ { “property”: “channel.featured_tab.template.string”, “value”: “Everything” }, { “property”: “channel.banner.mobile.medium.image.url”, “value”: “https://yt3.ggpht.com/-2yueuJ2GfNc/WSfJ67_xrYI/AAAAAAAAAqg/MIVb0xS9fUQA2Cn-ReWLpapK47WOVaPCQCL8B/w640-fcrop64=1,32b75a57cd48a5a8-nd-c0xffffffff-rj-k-no/channels4_banner” } ] } } “
As mentioned above, the process requires two separate API calls. We also have an existing png image channelbanner.png which is uploaded.
In the first API call to channelBanner.insert we ensure the following :
- Set cURL header to ‘Content-Type: application/octet-stream’ since we are sending the raw contents of the image
- Set cURL header to ‘Authorization: OAuth ‘ . $accessToken to pass the access token
- Set cURL header to ‘Content-Length: ‘ . filesize(“channelbanner.png”) to set the length of the data being posted.
On successful execution the response will return a json encoded ChannelBanner Resource. We extract the url property from there . This is the url of the channel banner.
We then execute the next API call to channel.update to set the banner image url. We define the required Channel resource classes and json encode the top level object. We set the url attribute to the value obtained from the previous API call.
We set the following things in the cURL request:
- Set header to Content-Type: application/json since we are sending the Channel object
- Set CURLOPT_CUSTOMREQUEST to PUT since this is a PUT request and not a POST
- Set CURLOPT_POSTFIELDS to the json encoded Channel object
On successful execution, the response contains a json encoded Channel resource object.
This is how the Channel banner looks after uploading channelbanner.png
Leave a Reply