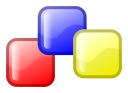
OVERVIEW
The wxTreeCtrl lets you display data in a hierarchical tree format. It supports different styles and has comprehensive events which can be processed. Given below is a sample tree which shows cities in countries.
SAMPLE CODE
tree.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif // WX_PRECOMP
#include <wx/treectrl.h>
class Tree: public wxFrame {
public:
Tree(const wxString &title);
private:
wxTreeCtrl *m_tree;
wxStaticText *m_title;
void treeSelChanged(wxTreeEvent &evt);
};
tree.cpp
#include "tree.h"
Tree::Tree(const wxString &title):
wxFrame(NULL, -1, title, wxDefaultPosition, wxSize(800,400)) {
wxPanel *panHome = new wxPanel(this, -1);
wxBoxSizer *vbox = new wxBoxSizer(wxVERTICAL);
panHome->SetSizer(vbox);
m_title = new wxStaticText(panHome, -1, wxT("Tree Demo"));
vbox->Add(m_title, 0, wxEXPAND | wxALL,2);
m_tree = new wxTreeCtrl(panHome, -1, wxDefaultPosition, wxDefaultSize, wxTR_HAS_BUTTONS | wxTR_SINGLE,
wxDefaultValidator, wxT("Tree Control"));
vbox->Add(m_tree, 4, wxEXPAND | wxALL, 2);
//populate tree
wxTreeItemId root = m_tree->AddRoot(wxT("Countries"), -1, -1, NULL);
wxTreeItemId uk = m_tree->AppendItem(root, wxT("United Kingdom"), -1, -1, NULL);
wxTreeItemId uk_london = m_tree->AppendItem(uk, wxT("London"), -1, -1, NULL);
wxTreeItemId uk_manchester = m_tree->AppendItem(uk, wxT("Manchester"), -1, -1, NULL);
wxTreeItemId uk_birmingham= m_tree->AppendItem(uk, wxT("Birmingham"), -1, -1, NULL);
wxTreeItemId usa = m_tree->AppendItem(root, wxT("United States"), -1, -1, NULL);
wxTreeItemId usa_ny = m_tree->AppendItem(usa, wxT("New York"), -1, -1, NULL);
wxTreeItemId usa_chi = m_tree->AppendItem(usa, wxT("Chicago"), -1, -1, NULL);
wxTreeItemId usa_det = m_tree->AppendItem(usa, wxT("Detroit"), -1, -1, NULL);
wxTreeItemId usa_wash = m_tree->AppendItem(usa, wxT("Washington"), -1, -1, NULL);
wxTreeItemId russia = m_tree->AppendItem(root, wxT("Russia"), -1, -1, NULL);
wxTreeItemId russia_moscow = m_tree->AppendItem(russia, wxT("Moscow"), -1, -1, NULL);
wxTreeItemId russia_lenin = m_tree->AppendItem(russia, wxT("Leningrad"), -1, -1, NULL);
wxTreeItemId italy = m_tree->AppendItem(root, wxT("Italy"), -1, -1, NULL);
wxTreeItemId italy_milan = m_tree->AppendItem(italy, wxT("Milan"), -1, -1, NULL);
wxTreeItemId italy_rome = m_tree->AppendItem(italy, wxT("Rome"), -1, -1, NULL);
wxTreeItemId italy_pisa = m_tree->AppendItem(italy, wxT("Pisa"), -1, -1, NULL);
m_tree->Bind(wxEVT_TREE_SEL_CHANGED, &Tree::treeSelChanged, this);
}
void Tree::treeSelChanged(wxTreeEvent &evt) {
wxTreeItemId item = evt.GetItem();
wxString lbl = m_tree->GetItemText(item);
wxMessageBox(lbl);
}
main.h
#include <wx/wxprec.h>
#ifndef WX_PRECOMP
#include <wx/wx.h>
#endif // WX_PRECOMP
#include "tree.h"
class MainApp: public wxApp {
public:
virtual bool OnInit();
};
main.cpp
#include "main.h"
bool MainApp::OnInit() {
Tree *tree = new Tree(wxT("Tree Demo App"));
tree->Show(true);
return TRUE;
}
wxIMPLEMENT_APP(MainApp);
The sample output is shown below:
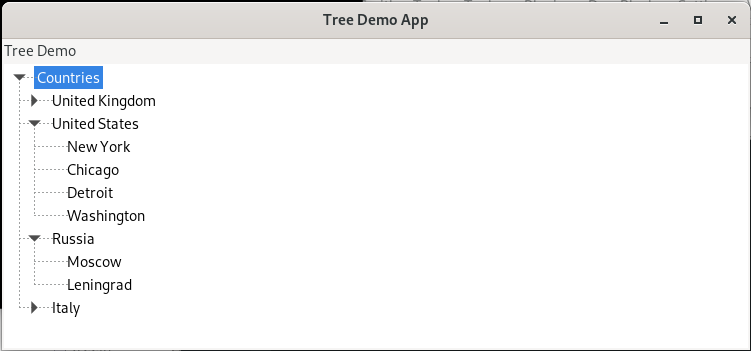
Leave a Reply