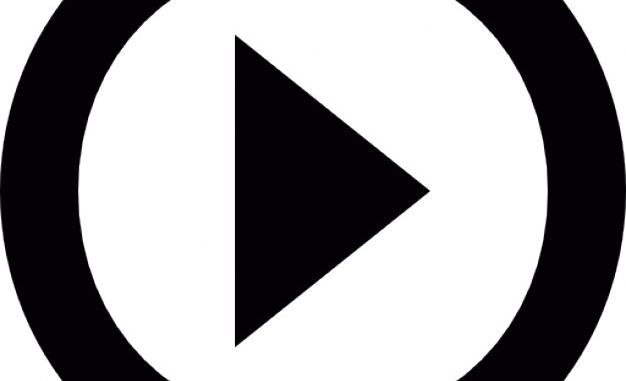
This is article 30 of the YouTube API With PHP series.
The delete function deletes a comment either posted by the current user, or posted to a Video or Channel owned by the current user. This call requires user-authentication.
Parameters
- key (string) required. Your API key
- access_token (string) required in certain cases. This is the user Access token.
- id (string). Required. Id of the comment.
Example Requests
Delete a comment.
https://www.googleapis.com/youtube/v3/comments?key=xx&access_token=xx&id=xx
Response
On successful execution this method returns an HTTP 204 response code (No Content). If you specify a non-existent comment id then the call will fail.
Sample code to delete a comment:
<?php error_reporting(E_ALL ^ E_NOTICE ^ E_WARNING ^ E_DEPRECATED); set_time_limit(60 * 3); session_start(); $g_youtubeDataAPIKey = "***"; $commentId = "z12jy3qp3q21e3se322ttjmaqlimxxohg04.1496042388885297"; $_SESSION["code_id"] = $_SERVER["PHP_SELF"]; if ($_SESSION["access_token"] == null || $_SESSION["access_token"] == "") { // check for oauth response header("Location: ../../init-login.php"); exit; } $accessToken = $_SESSION["access_token"]; // make api request $url = "https://www.googleapis.com/youtube/v3/comments?key=" . $g_youtubeDataAPIKey . "&access_token=" . $accessToken . "&id=" . $commentId; $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_RETURNTRANSFER => 1, CURLOPT_URL => $url, CURLOPT_USERAGENT => 'YouTube API Tester', CURLOPT_SSL_VERIFYPEER => 1, CURLOPT_SSL_VERIFYHOST=> 0, CURLOPT_HEADER=>1, CURLOPT_CAINFO => "../../cert/cacert.pem", CURLOPT_CAPATH => "../../cert/cacert.pem", CURLOPT_FOLLOWLOCATION => TRUE, CURLOPT_CUSTOMREQUEST=>"DELETE" )); $resp = curl_exec($curl); curl_close($curl); var_dump($resp); ////////////////////////////////// class Comment { public $snippet; public $id; } class Snippet { public $textOriginal; } ?>
Here is the output:
string(344) “HTTP/1.1 204 No Content Cache-Control: no-cache, no-store, max-age=0, must-revalidate Pragma: no-cache Expires: Mon, 01 Jan 1990 00:00:00 GMT Date: Mon, 29 May 2017 05:22:36 GMT ETag: “m2yskBQFythfE4irbTIeOgYYfBU/7MMpT8ZDo9n_QH3wl-DdyiOMn04″ Vary: Origin Vary: X-Origin Server: GSE Alt-Svc: quic=”:443″; ma=2592000; v=”38,37,36,35″ “
Leave a Reply