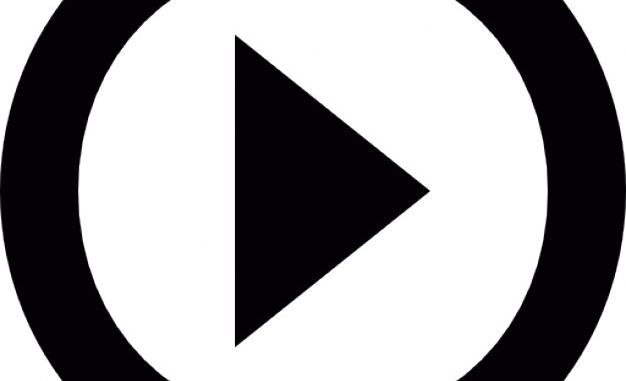
This is article 21 of the YouTube API With PHP series.
The delete function deletes an existing ChannelSection. This call requires user-authentication so only ChannelSections belonging to the current user can be deleted.
The Request URL is
DELETE https://www.googleapis.com/youtube/v3/channelSections
Parameters:
- key (string) required. Your API key
- access_token (string) required. This is the user Access token.
- id (string) required. A valid ChannelSection id
- onBehalfOfContentOwner (string) optional. This is relevant only for YouTube Channel Partners. For this parameter, the API request URL should have user authentication.We will not be exploring this option.
Example Requests
Delete a ChannelSection
https://www.googleapis.com/youtube/v3/channelSections?key=xx &access_token=xx &id=xxxxx
Response
On successful execution, a 204 http header will be received.
Sample code to delete a ChannelSection
<?php error_reporting(E_ALL ^ E_NOTICE ^ E_WARNING ^ E_DEPRECATED); set_time_limit(60 * 3); session_start(); $g_youtubeDataAPIKey = "**"; $channelId = "UCo6DJdltbIub80bLiyJRv3w"; $_SESSION["code_id"] = $_SERVER["PHP_SELF"]; if ($_SESSION["access_token"] == null || $_SESSION["access_token"] == "") { // check for oauth response header("Location: ../../init-login.php"); exit; } $accessToken = $_SESSION["access_token"]; // make api request $url = "https://www.googleapis.com/youtube/v3/channelSections?key=" . $g_youtubeDataAPIKey . "&access_token=" . $accessToken . "&id=UCnXmfpAZ1rLsg0Goh0bBHUA.LeAltgu_pbM"; $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_RETURNTRANSFER => 1, CURLOPT_URL => $url, CURLOPT_USERAGENT => 'YouTube API Tester', CURLOPT_SSL_VERIFYPEER => 1, CURLOPT_SSL_VERIFYHOST=> 0, CURLOPT_HEADER=>1, CURLOPT_CAINFO => "../../cert/cacert.pem", CURLOPT_CAPATH => "../../cert/cacert.pem", CURLOPT_FOLLOWLOCATION => TRUE, CURLOPT_CUSTOMREQUEST=>"DELETE", )); $resp = curl_exec($curl); curl_close($curl); var_dump($resp); ?>
Here is the output:
string(344) “HTTP/1.1 204 No Content Cache-Control: no-cache, no-store, max-age=0, must-revalidate Pragma: no-cache Expires: Mon, 01 Jan 1990 00:00:00 GMT Date: Sat, 27 May 2017 07:03:19 GMT ETag: “m2yskBQFythfE4irbTIeOgYYfBU/7MMpT8ZDo9n_QH3wl-DdyiOMn04″ Vary: Origin Vary: X-Origin Server: GSE Alt-Svc: quic=”:443″; ma=2592000; v=”38,37,36,35″ “
The following things are required in the cURL Post:
- Put CURLOPT_HEADER as 1 since the response will return only headers
- Set CURLOPT_CUSTOMREQUEST to “DELETE” since this is not a POST
Leave a Reply